How to Escape Character Sequences on a String in MATLAB
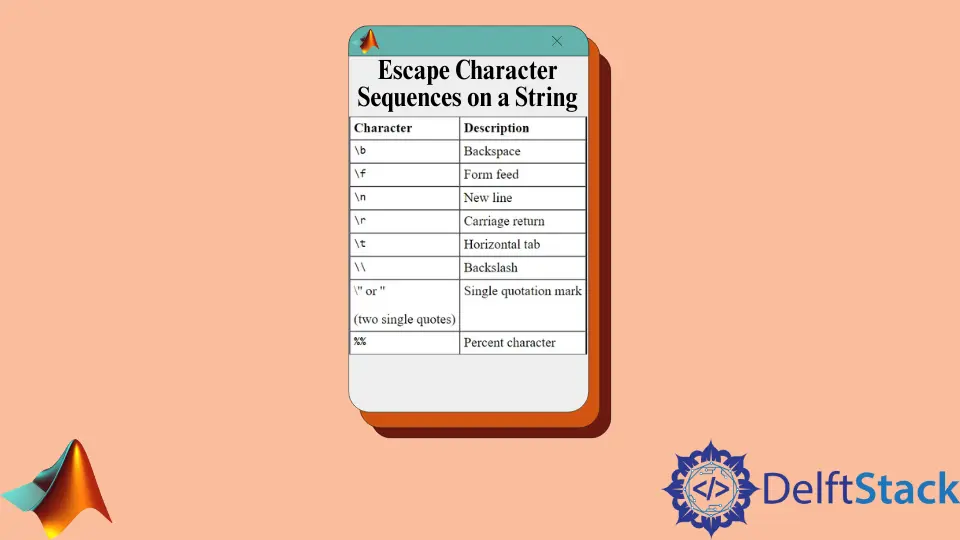
In the world of programming, handling strings effectively is crucial, especially when it comes to special characters. In MATLAB, strings can contain characters that have specific meanings, such as quotation marks, backslashes, and newlines. To manage these characters without causing errors or unexpected behavior, we use escape character sequences.
This tutorial aims to demonstrate how to utilize these sequences in MATLAB strings, ensuring your code runs smoothly and as intended. Whether you’re a beginner or an experienced coder, understanding string manipulation can greatly enhance your MATLAB skills. Let’s dive into the various methods to escape character sequences in MATLAB strings.
Understanding Escape Character Sequences
Before we jump into the methods of escaping character sequences, it’s important to understand what they are. In programming, an escape character is a character that invokes an alternative interpretation on subsequent characters in a string. In MATLAB, the backslash (\
) serves as the escape character. This means that when you want to include a special character in a string, you precede it with a backslash to signify that it should be treated literally.
For example, to include a double quote in a string, you would write it as \"
. This allows MATLAB to recognize that you want the quotation mark to be part of the string rather than signaling the end of the string.
Using Escape Sequences in Strings
MATLAB offers a straightforward way to include escape sequences in your strings. Here are some common escape sequences you might encounter:
\'
- Single quote\"
- Double quote\\
- Backslash\n
- New line\t
- Horizontal tab
Let’s look at how to implement these escape sequences in a MATLAB string.
Example: Including Quotes in a String
When you want to include quotes in a string, you can escape them using the backslash. Here’s how you do it:
str = 'She said, \"Hello, World!\"';
disp(str);
Output:
She said, "Hello, World!"
In this example, the backslashes before the double quotes allow MATLAB to include the quotes in the output string. Without the escape character, MATLAB would interpret the quotes as the start and end of the string, leading to an error.
Example: Including New Lines and Tabs
You can also use escape sequences to format your strings. For instance, if you want to add a new line or a tab, you can do so as follows:
str = 'Hello,\nWelcome to MATLAB!\nEnjoy coding!\n\t- Your friendly assistant';
disp(str);
Output:
Hello,
Welcome to MATLAB!
Enjoy coding!
- Your friendly assistant
In this example, the \n
escape sequence creates new lines in the output, while the \t
adds a tab space before the last line. This makes the output more readable and organized.
Example: Using Backslashes in Strings
Backslashes can be tricky since they are also used as escape characters. To include a literal backslash in your string, you need to escape it as well:
str = 'This is a backslash: \\';
disp(str);
Output:
This is a backslash: \
Here, the double backslash \\
is interpreted as a single backslash in the output. This is a common requirement in many programming tasks, especially when dealing with file paths.
Conclusion
Mastering escape character sequences in MATLAB is essential for effective string manipulation. By understanding how to use the backslash to escape special characters, you can ensure that your strings are formatted correctly and that your code runs without errors. Whether you’re adding quotes, new lines, or backslashes to your strings, these techniques will enhance your programming skills and make your code more robust. Keep practicing these methods, and soon you’ll be handling strings in MATLAB like a pro!
FAQ
-
What is an escape character in MATLAB?
An escape character in MATLAB is a special character that alters the interpretation of subsequent characters in a string, with the backslash () being the most common. -
How do I include a single quote in a MATLAB string?
You can include a single quote in a MATLAB string by preceding it with a backslash, like this: ' .
-
Can I use escape sequences in string arrays?
Yes, escape sequences can be used in string arrays just like in regular character arrays in MATLAB. -
What happens if I forget to escape a special character?
If you forget to escape a special character, MATLAB may misinterpret your string, leading to syntax errors or unexpected output. -
Are escape sequences the same in all programming languages?
No, escape sequences can vary between programming languages, so it’s important to refer to the specific language documentation for details.
Mehak is an electrical engineer, a technical content writer, a team collaborator and a digital marketing enthusiast. She loves sketching and playing table tennis. Nature is what attracts her the most.
LinkedIn