How to Comment Multiple Lines in MATLAB
- Commenting Multiple Lines of Code Using the Comment Block in MATLAB
- Commenting Multiple Lines of Code Using the Editor in MATLAB
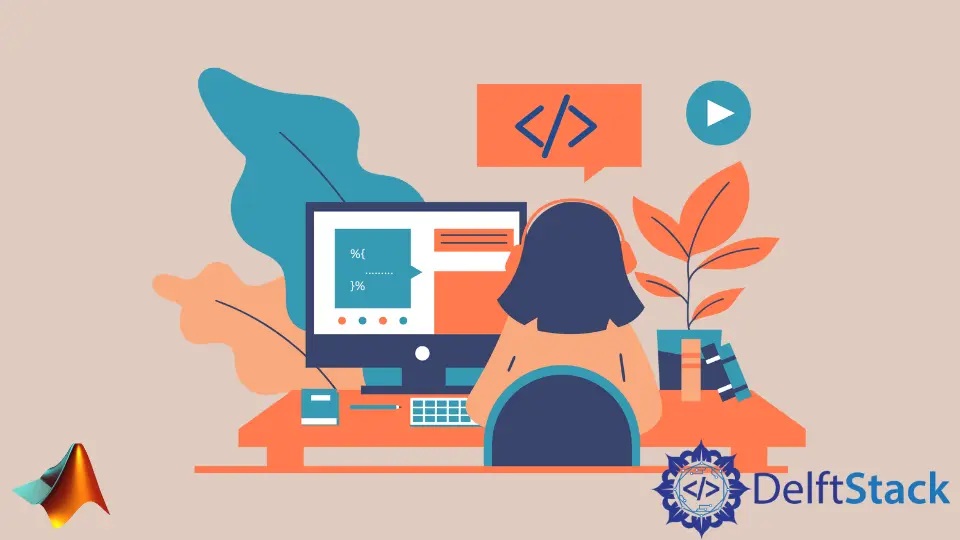
This tutorial will discuss how to comment multiple lines of code in MATLAB using the comment block method and MATLAB editor.
Commenting Multiple Lines of Code Using the Comment Block in MATLAB
To comment a single or two lines of code, we can use the %
character to do that. But if we have to comment multiple lines of code, this method will take a lot of time. Instead of using %
to comment lines, we can use the comment block to comment multiple lines of code. Anything which is written inside this block will be treated as a comment. See the example code below.
matlabCopy%{
comments
}%
In the above code, everything written inside these brackets will be treated as a comment. You can increase the block as you like.
Commenting Multiple Lines of Code Using the Editor in MATLAB
There is another way of commenting multiple lines of code at once using the MATLAB editor. You have to select all the lines you want to comment and then from the MATLAB editor, select the option comment
to comment all the selected lines of code. If you want to uncomment multiple lines, select all the lines you want to uncomment and select the option uncomment
from the MATLAB editor to uncomment all the selected code lines. You can also use the shortcuts for these options.