How to Convert ASCII to String in MATLAB
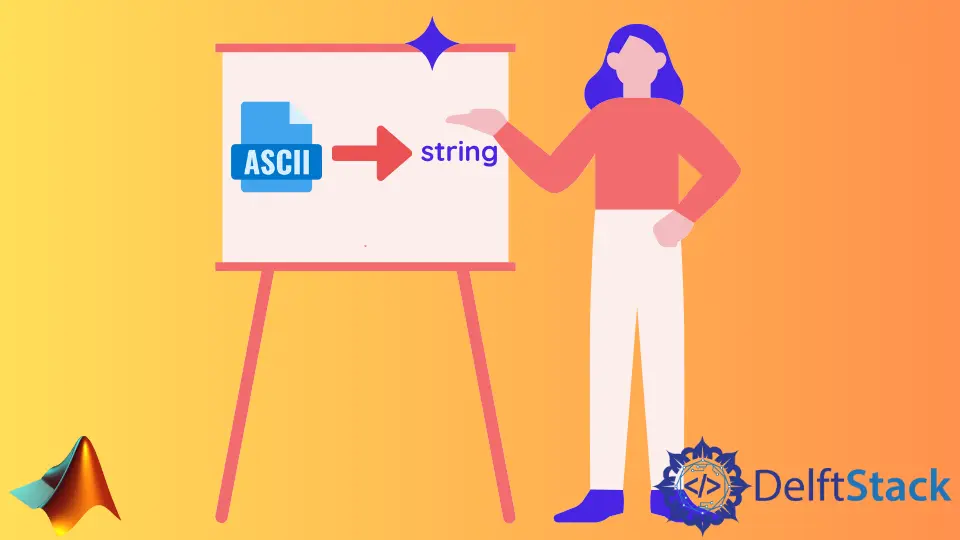
This tutorial will introduce how to convert the ASCII value to a string using the char()
function in MATLAB.
Convert the ASCII Value to String Using the char()
Function in MATLAB
ASCII code is used to represent characters. Every character has a unique ASCII value, for example, the character a
has an ASCII value of 97, and character b
has an ASCII value of 98, and so on. In MATLAB, if you have ASCII values of some characters and want to convert them into their equivalent string or character, you can do that using the char()
function. For example, let’s convert some ASCII values to their equivalent characters or string. See the code below.
ascii_values = [97 98 100]
characters = char(ascii_values)
Output:
ascii_values =
97 98 100
characters =
'abd'
As you can see in the output, the ASCII values are converted into their equivalent characters or string. If you want to convert characters or strings into their equivalent ASCII values, you can use the double()
function in MATLAB. For example, let’s convert the above characters or strings to their equivalent ASCII values. See the code below.
characters = 'abd'
ascii_values = double(characters)
Output:
characters =
'abd'
ascii_values =
97 98 100
As you can see in the output, we converted the characters or string into their equivalent ASCII values using the double()
function in MATLAB.