How to Use Z Flag in Linux Bash
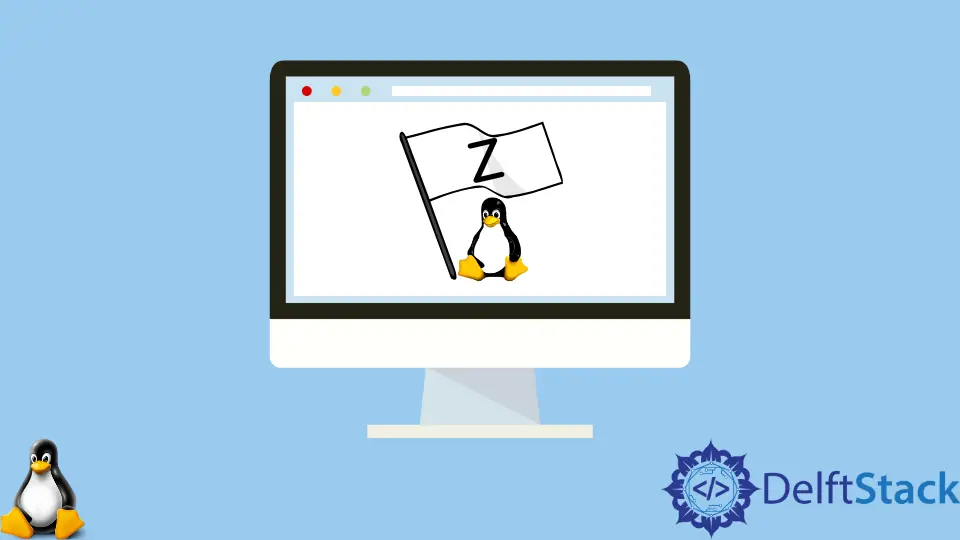
Bash is a powerful scripting language for creating programs on Linux. Like every programming language, we can make comparisons with Bash scripting.
This article will explain the test
command used for comparison and the -z
flag parameter used with the test
command in Linux Bash.
Comparison With the test
Command in Bash
The test
command is a built-in command in Linux used to check file types and compare values. With this command, we can perform comparison operations in Bash scripting.
Simple usage is as follows.
test 2 -gt 1 && echo "2 is greater" || echo "1 is greater"
The comparison expression is after the test
command. Then there are the operations we want to do depending on whether the result is true
or false
.
We can also use the test
command as [ ]
. The example below is the same as above.
[ 2 -gt 1 ] && echo "2 is greater" || echo "1 is greater"
Use the -z
Flag in Bash
The test
command can be used with a parameter. The -z
flag is a parameter that checks if the length of a variable is zero and returns true
if it is zero.
In the example below, the -z
flag is used with the test
command, and it is tested whether the given string is empty.
[ -z "" ] && echo "empty" || echo "non-empty"
[ -z "test string" ] && echo "empty" || echo "non-empty"
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn