Ternary Operator in Bash Script
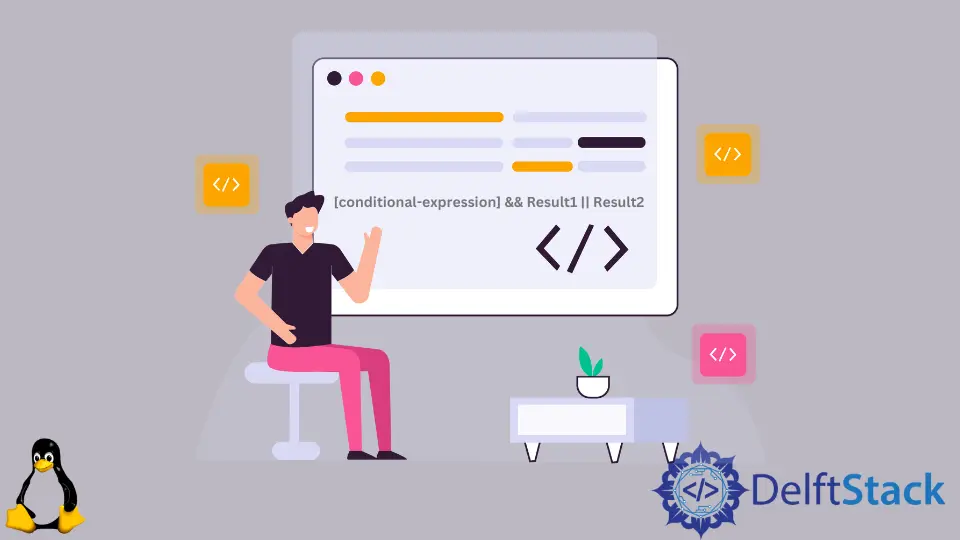
This article is a trivial guide to the conditional operator, also known as the ternary operator, in Bash script.
Ternary Operator in Bash Script
The ternary or conditional operator is usually used as an in-line replacement of the if..else
statement. In most programming languages, it uses two symbols ?
and :
to make a conditional statement.
A common syntax for the ternary conditional operator:
ReturnValue = expression ? trueValue : falseValue
Bash doesn’t have direct support for the conditional operator. However, this ternary operation can be achieved using the following conditional statement.
[conditional-expression] && Result1|| Result2
This expression is evaluated as if the conditional-expression
is true
, then the &&
operator will be operated, and the Result1
will be the answer. But if the conditional-expression
is false
, then the second logical operator ||
will run, and it will give Result2
as an answer.
Script:
#!/bin/bash
echo "Enter Your Age: "
read a;
[[ $a == 25 ]] && res="yes" || res="no"
echo "Elgibility: $res" ;
Output:
We have run the program twice from the output to get both results.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn