How to Replace Strings in Bash
- Replace One Substring for Another String in Bash
- Replace the Entire String Equal to Substring
- Replace the Last Substring Detected in a String in Bash
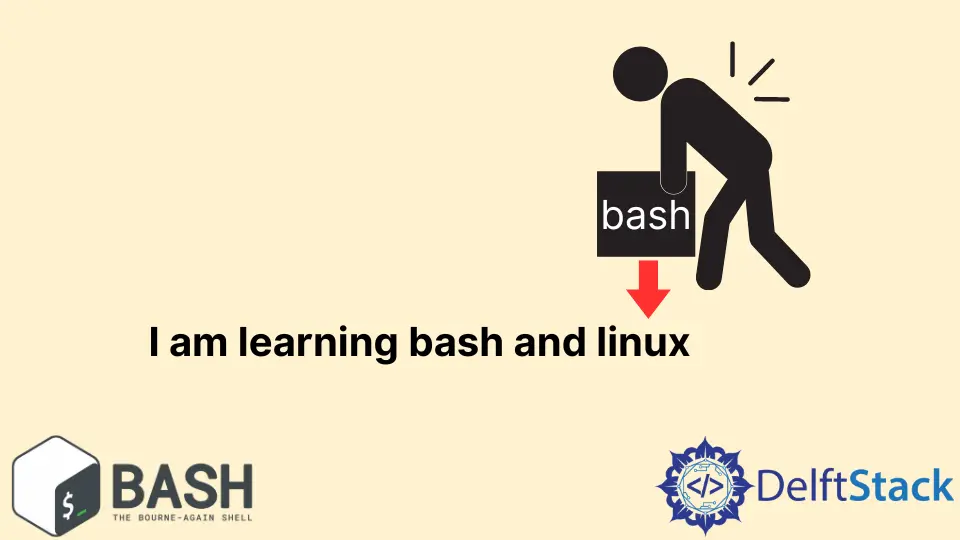
Bash allows us to make calls to other programs, and by specifying the necessary inputs and outputs, we may generate any outcome. This article will introduce how to replace one substring with another string.
Replace One Substring for Another String in Bash
The command below will replace the first string discovered that matches a substring starting from the first character of the string with a replacement string.
${string/substring/replacement}
Let’s see an example.
$ text="I am learning linux and linux"
$ reptext="bash"
$ echo "${text/linux/"$reptext"}''
Output:
I am learning bash and linux
As mentioned above, the first string linux
is replaced by bash
, and the other one is untouched.
Replace the Entire String Equal to Substring
We use the command below to replace the entire string equal to the substring with the replacement string.
${string//substring/replacement}
Let’s see an example:
$ text="I am learning linux and linux"
$ reptext="bash"
$ echo "${text//linux/"$reptext"}"
Output:
I am learning bash and bash
Now there are two slashes (//)
instead of one(/)
. In the output, we can see that the above command replaced all substrings equal to the replacement string.
Replace the Last Substring Detected in a String in Bash
We will use the command bellow to replace the last substring detected in a string with a replacement string.
${string/%substring/replacement}
Let’s see an example:
$ text="I am learning linux and linux"
$ reptext="bash"
$ echo "${text/%linux/"$reptext"}"
Output:
I am learning linux and bash