How to Check if String Contains a Substring in Bash
-
Use the
case
Conditional Statement (Method 1) -
Use Wildcards in the
if
Statement (Method 2) -
Use the
grep
Command of Bash (Method 3) -
Use the Regex Operator (
~=
) (Method 4)
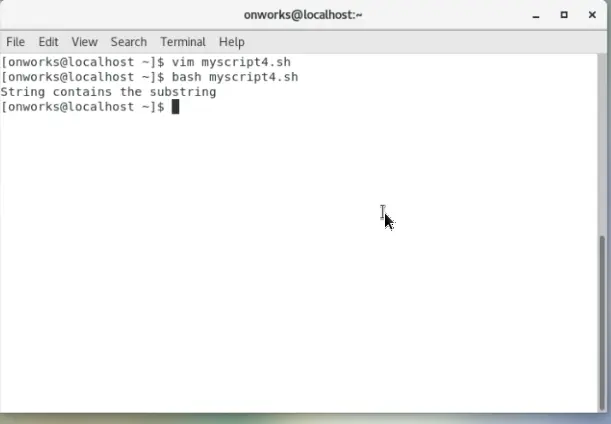
Finding substring from a string is the most frequently used operation with strings. There are many ways to perform this task.
In this article, we will see multiple Bash script-based implementations to find if a given string contains a particular substring or not.
Use the case
Conditional Statement (Method 1)
The case
is a conditional statement in bash and can be used to implement a conditional block in the script. This statement can be used to find a substring in bash.
Script:
#!/bin/bash
str='This is a bash tutorial'
substr='bash'
case $str in
*"$substr"*)
echo "str contains the substr"
;;
esac
We have 2 strings, str
and substr
. We have applied a case
statement to find if str
contains substr
or not.
Output:
Use Wildcards in the if
Statement (Method 2)
We can also find a substring from a string using wildcards in the if
statement. The simplest way to find a substring is by putting the wildcard symbol asterisk (*
) around the substring and comparing it with the actual string.
Script:
#!/bin/bash
str='This is a bash tutorial'
substr='tutorial'
if [[ "$str" == *"$substr"* ]]; then
echo "String contains the sunstring"
else
echo "String does'nt contains the substring"
fi
Output:
Use the grep
Command of Bash (Method 3)
grep
command is also used to find something from a file or a string. It has an option -q
, which tells the grep
command to not display the output; return true
or false
.
Script:
#!/bin/bash
str='This is a bash tutorial'
substr='tutorial'
if grep -q "$substr" <<< "$str"; then
echo "String contains the substring"
fi
Output:
Use the Regex Operator (~=
) (Method 4)
There is another operator known as regex operator
(~=) with which we can compare two strings and whether a string contains a substring.
Script:
#!/bin/bash
str='This is a bash tutorial'
substr='bash'
if [[ "$str" =~ .*"$substr".* ]]; then
echo "String contains the substring"
fi
Note that in the conditional statement, the regex operator makes the right-hand side string a regular expression and the symbol .*
means to compare 0 or more occurrences of a substring in the string.
Output:
Hence, you can see several ways to find a substring from a string.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn