How to Solve Unary Operator Expected Error in Bash
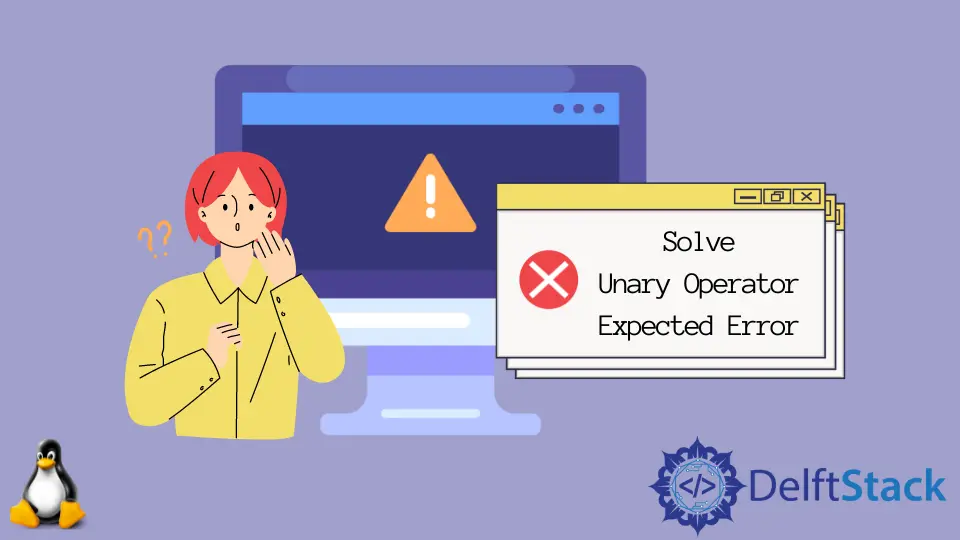
In shell scripting, the unary operator operates on a single operand to return a new value. This article will explain how to solve the [: =: unary operator expected
error in Linux Bash.
Solve the [: =: unary operator expected
Error in Bash
Suppose your bash script specifies a line containing a binary operator that does not apply to two arguments. Bash assumes that you will use a unary operator and get the [: =: unary operator expected
error.
We will examine the causes and solutions for this error in detail in the rest of the article.
Let’s save the bash script below as file.sh
and try to execute it with the bash file.sh
command.
Command:
#!/bin/bash
str=
if [ $str == "string" ]; then
echo "Go on!"
fi
The code will not execute and generates an error. The unassigned variable "str"
is used with single square brackets.
Execute the file again with the bash -x file.sh
command to debug it.
Output:
As you see, there is no value on the left side of the equation, and this causes the error.
If you write the variable in double quotes, you will not get this error. Because now the comparison operation will become '' = string
.
Having values on both sides of the operator ensures that the code will run correctly.
Command:
#!/bin/bash
str=
if [ "$str" == "string" ]; then
echo "Go on!"
fi
Another way to solve the error is to use double square brackets. The comparison operation will also be '' = string
in this way.
Command:
#!/bin/bash
str=
if [[ $str == "string" ]]; then
echo "Go on!"
fi
If you want to see if a variable is empty, you can use the -z
flag instead of comparing. The -z
flag checks if the length of the variable is zero, and it returns true
if it is zero.
Command:
#!/bin/bash
str=
if [ -z $str ]; then
echo "It is empty string"
fi
This article describes general solutions. By debugging your code, you can find the variables that cause the error and solve it as explained.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedInRelated Article - Bash Operator
- How to Use Double and Single Pipes in Bash
- How to Use the Mod Operator in Bash
- Ternary Operator in Bash Script
- Logical OR Operator in Bash Script
- The -ne Operator in Bash