How to Use the shift Command in Bash Scripts
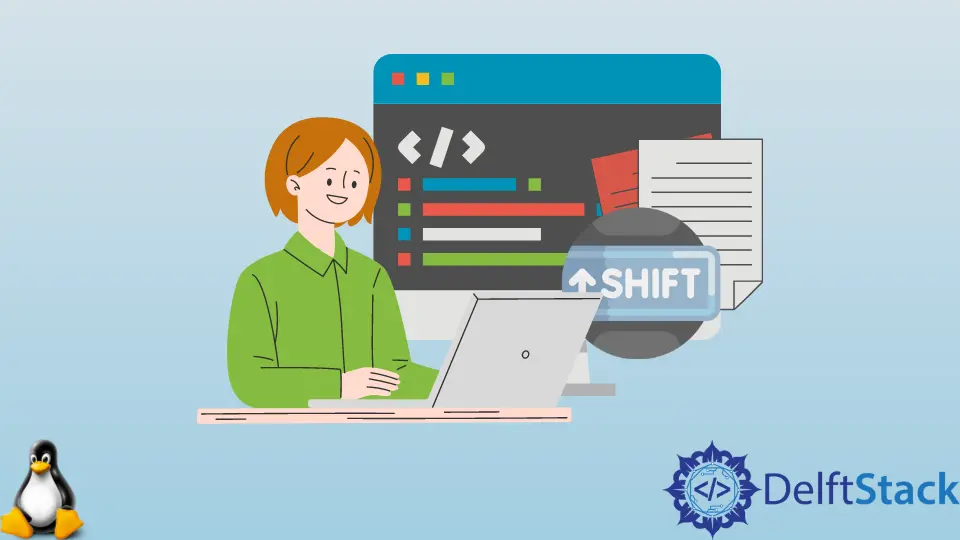
In Unix-like operating systems, the shift
command is a built-in command in the Bash shell that makes the indexing of arguments start from the shifted position, N
. The command removes the first argument in the list.
The shift
command takes only a single argument, an integer
.
Using the shift
Command in Bash Script
The bash script below demonstrates using the shift
command. The bash script has an add
function, and inside the function, we have three sections.
Each section adds two numbers and prints the sum. The last line of the bash script calls the function and passes 5
arguments to the function.
Example:
#!/bin/bash
add(){
echo "Using default shift value"
sum=`expr $1 + $2`
echo \$1=$1
echo \$2=$1
echo "sum="$sum
echo "------------"
echo "Passing the integer,1, to the shift command"
shift 1
sum1=`expr $1 + $2`
echo \$1=$1
echo \$2=$2
echo "sum="$sum1
echo "------------"
echo "Passing the integer,2, to the shift command"
shift 2
sum3=`expr $1 + $2`
echo \$1=$1
echo \$2=$2
echo "sum="$sum3
}
add 1 2 3 4 5
Output:
The first section of the add
function does not use the shift command.
From the bash script above, the variable $1
will take 1
and $2
will take 2
. The sum of the numbers will be 3
.
The second section passes the integer 1
to the shift
command. We ignore the first argument being passed to the function, as the first argument will be the second argument, the next argument will be the third argument, and so on.
The variable $1
will take the value 2
, and the variable $2
will take 3
. The sum of the values of the variables is 5
.
The last section passes the integer, 2
to the shift
command. We ignore 2
and 3
in the second and third arguments.
We will start counting the first argument as 4
and the second argument as 5
. The sum of the two arguments is 9
.