shebang in Bash Script
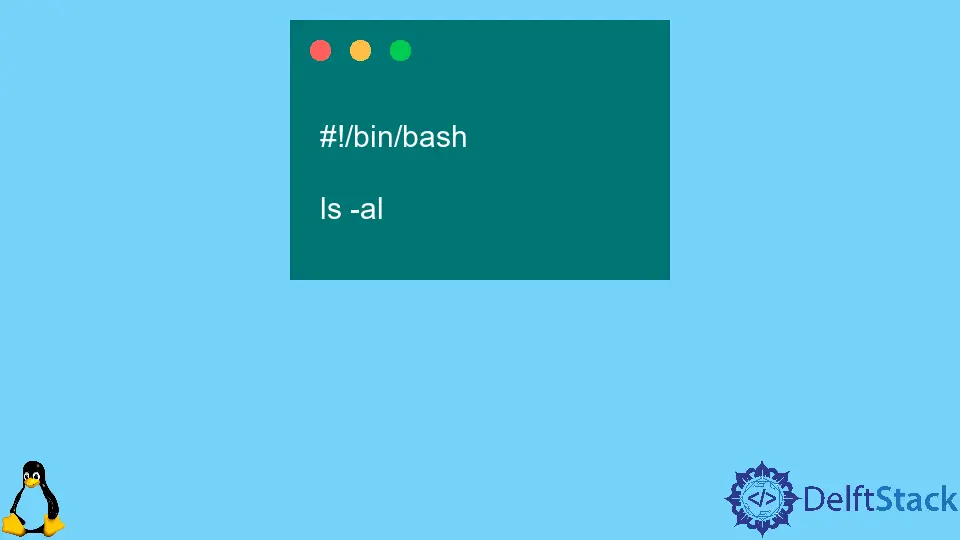
This tutorial explains what the shebang is and its usage in bash scripts.
Bash Shebang (#!
) and How to Use It
The #!
syntax is used in shell scripts to indicate the interpreter to execute the script in Unix/Linux operating systems.
#!/bin/bash
The shebang starts with the #
sign and an exclamation mark followed by the full path to the interpreter.
In the case of the code snippet given above, it specifies that the script should be executed using bash
as the interpreter, and the bash
interpreter can be found in the /bin
directory. We assume that bash
has been installed in the /bin
directory.
Scripts in Linux are executed by the interpreter specified on the first line.
Let us write a script that uses #!/bin/bash
. The script below prints all the content of the folder in a long format using bash
as its interpreter.
#!/bin/bash
ls -al
Make Linux Scripts Portable
#!/bin/bash
assumes that bash is installed in the /bin
directory. However, this is not always the case across different Unix
like operating systems. To make scripts more portable, use #!/usr/bin/env bash
.
#!/usr/bin/env bash
This tells the shell to search for the first match of bash
in the $PATH
variable and use the first one it finds as the interpreter. This is useful if you are not aware of the absolute path of the interpreter on the system.
Let us write a script that uses #!/usr/bin/env bash
. The script below prints all the folder’s content in a long format using the first bash
shell it finds in the $PATH
variable as its interpreter.
#!/usr/bin/env bash
ls -al