How to Find and Replace Complex Strings by Using Sed With Regex
-
Find and Replace Strings Using
sed
in Bash -
Find and Replace the Delimiter Character
/
in a String in Bash - Find All the Numbers and Replace Them With the String Number in Bash
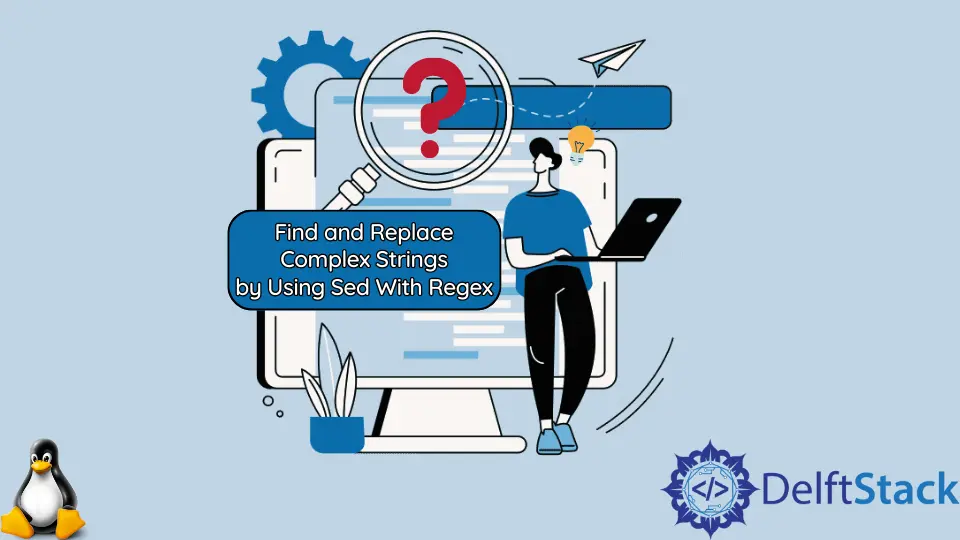
When working with text files, you’ll frequently need to identify and replace text strings in one or more files.
sed
is a stream editor. It can perform rudimentary text manipulation on files and input streams such as pipelines. You can use sed
to search for, find, and replace words and lines, as well as insert and delete them.
Basic and extended regex
is supported, allowing you to match complex patterns. In this post, we’ll look at how to use sed
to find and replace strings. We’ll also demonstrate how to use recursive search and replace.
Find and Replace Strings Using sed
in Bash
There are various versions of sed
, each with its features.
Linux distributions come with GNU sed
pre-installed by default, but Mac OS utilizes the BSD version. We’re going to use the GNU
version.
The syntax is used for searching and replacing text with sed
:
sed -e 's/Search_Regex/substitution/g' Filename
In the above syntax used, the following are the definition:
- The
-e
is sed’s output written to the standard output by default. This parameter instructssed
to edit files in their current location. If an extension is defined, a replacement file is generated. - The
///
is a character that acts as a separator. Although most people use the slash (/
) character, it can be any character. - The
Search_Regex
is a to search for something. We will be using aregex
or a regular string. - The
substitution
is used as strings to substitute. - The
g
is a flag for global substitution. Thesed
reads the file line by line by default and only alters the first occurrence of theSearch_Regex
on each line. All instances are replaced when the replacement flag is set. - The
Filename
is the file’s name that should run the command.
Let’s look at the commonly used parameters and flags for using the sed
command to search and replace text in files. We will use the example file we have created for demonstration purposes, i.e., live.txt
.
live.txt
Omicron is the endgame of Covid and Omicron 9876
If the g
flag is ignored, only the first instance of the search string in each line is replaced.
$ sed -e 's/endgame/infinitywar/' live.txt
Output:
Omicron is the infinitywar of Covid and Omicron 9876
The sed
replaces all occurrences of the search pattern with the global replacement flag:
$ sed -e 's/Omicron/thanos/g' live.txt
Output:
thanos is the endgame of Covid and thanos 9876
As you may have observed, the string Omicron
has been substituted with thanos
.
Find and Replace the Delimiter Character /
in a String in Bash
You must use the backslash \
to escape the slash if you want to discover and replace a string that contains the delimiter character /
. For example, to replace /bin/bash
with /usr/bin/zsh
, use the following:
$ sed -e 's/\/bin\/bash/\/usr\/bin\/zsh/g' live.txt
Output:
Omicron is the endgame of Covid and Omicron 9876
Let’s make it easier to understand using a vertical bar |
or a colon :
, although any other character will suffice.
$ sed -e 's|/bin/bash|/usr/bin/zsh|g' live.txt
Output:
Omicron is the endgame of Covid and Omicron 9876
As you can see, the result is identical to the prior one.
Find All the Numbers and Replace Them With the String Number in Bash
Regular expressions can also find all four numbers and replace them with the string number. For example:
$ sed -e 's/\b[0-9]\{4\}\b/number/g' live.txt
Output:
Omicron is the endgame of Covid, and Omicron number
As you can see, the 9876
is replaced by the number
string.
Last but not least, whenever you use sed
to edit a file, you should always make a backup. Give the -i
option an extension for the backup file to accomplish this.
For example, edit live.txt
and save the original file as live.txt
. If you were to utilize bak
, you’d do that by:
$ sed -i.bak 's/Omicron/thanos/g' live.txt
List the files with the ls
command to ensure that the backup was created:
$ ls
Output:
live.txt
live.txt.bak
Although it may appear complicated at first, finding and changing the text in files with sed
is relatively straightforward.