How to Search for Files With a Filename Beginning With a Specified String in Bash
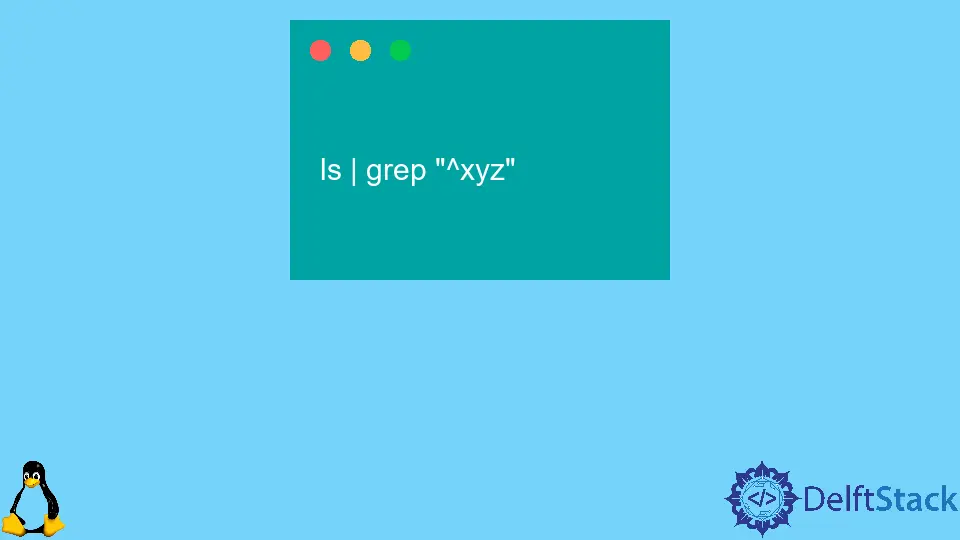
In this article, we will learn about finding all files with a filename beginning with a specified string.
Search for Files With a Filename Beginning With a Specified String in Bash
Use grep
Command
In Linux, the grep
command filters the search made within a file for a specific pattern of characters. It is one of the most often used commands in the Linux tool suite, and its purpose is to display the lines in the file that contain the pattern we are looking for.
The term regular expression
will be used to refer to the pattern we are attempting to search for within the file.
Syntax:
grep [options] pattern [files]
Example:
ls | grep "^xyz"
The command above returns all files that begin with the substring "xyz"
. It only works on the directory that you are now in
Use find
Command
The find
command works its way down into subfolders. To search for files that start with your string, use the following command.
find . -name 'xyz*'