How to Run GDB in Bash Script
- Understanding GDB and Bash Scripts
- Setting Up Your Environment
- Running GDB in a Bash Script
- Debugging with Breakpoints
- Automating GDB Commands
- Conclusion
- FAQ
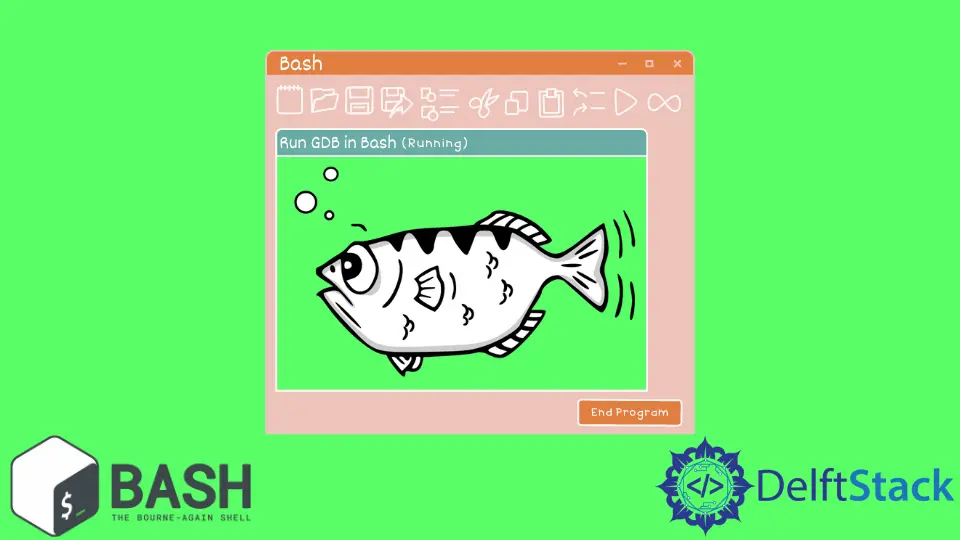
Running GDB (GNU Debugger) in a Bash script can streamline your debugging process, especially when dealing with binary files that require command-line arguments.
This tutorial will guide you through the steps to effectively incorporate GDB into your Bash scripts. Whether you are debugging complex applications or simple programs, integrating GDB can save you time and make your debugging sessions more efficient. By the end of this article, you’ll have a solid understanding of how to set up and run GDB within a Bash script, enabling you to debug your applications seamlessly.
Understanding GDB and Bash Scripts
Before diving into the practical aspects, let’s clarify what GDB and Bash scripts are. GDB is a powerful debugger for programs written in languages like C and C++. It allows developers to inspect what is happening inside a program while it executes or to analyze the program after it crashes. Bash scripts, on the other hand, are files containing a series of commands that the Bash shell can execute. Combining these two tools can significantly enhance your debugging capabilities.
Setting Up Your Environment
To start, ensure you have GDB installed on your system. You can check this by running the following command in your terminal:
gdb --version
If GDB is not installed, you can install it using your package manager. For example, on Ubuntu or Debian, you can run:
sudo apt-get install gdb
Once you have GDB installed, you can create a simple Bash script to run your GDB session. Let’s create a sample binary file to work with. For this example, we’ll use a simple C program that takes command-line arguments.
Sample C Program
#include <stdio.h>
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("No arguments provided.\n");
return 1;
}
printf("Argument: %s\n", argv[1]);
return 0;
}
Compile this code into a binary file named example
:
gcc -o example example.c
Now that we have our binary, we can proceed to run GDB in a Bash script.
Running GDB in a Bash Script
You can create a Bash script that runs GDB with your binary file. Here’s a basic example of how to do this:
#!/bin/bash
gdb -ex "run arg1" -ex "bt" --args ./example arg1
Make sure to give your script executable permissions:
chmod +x your_script.sh
Now, let’s break down the script. The -ex
option allows you to specify commands that GDB should execute. In this case, we’re telling GDB to run the program with arg1
and then print the backtrace (bt
) if the program crashes. The --args
option is used to pass the arguments to the binary.
Output:
Starting program: /path/to/example arg1
Argument: arg1
[Inferior 1 (process 1234) exited with code 0]
This output indicates that the program ran successfully with the provided argument.
Debugging with Breakpoints
One of the most powerful features of GDB is the ability to set breakpoints. You can modify your Bash script to include breakpoints for more granular debugging.
Here’s how you can set a breakpoint in your script:
#!/bin/bash
gdb -ex "break main" -ex "run arg1" -ex "bt" --args ./example arg1
In this script, we added a breakpoint at the main
function. When you run this script, GDB will pause execution at main
, allowing you to inspect variables and program state before continuing.
Output:
Breakpoint 1, main (argc=2, argv=0x7fffffffe3b8) at example.c:5
5 if (argc < 2) {
This output shows that GDB has successfully hit the breakpoint, allowing you to examine the state of the program at that moment.
Automating GDB Commands
You can further automate your debugging process by creating a GDB command file. This file can contain a series of commands that GDB will execute when it starts.
Creating a GDB Command File
First, create a file named gdb_commands.txt
with the following content:
break main
run arg1
bt
Now, modify your Bash script to use this command file:
#!/bin/bash
gdb -x gdb_commands.txt --args ./example arg1
This approach keeps your script clean and allows you to easily modify GDB commands without changing the Bash script itself.
Output:
Breakpoint 1, main (argc=2, argv=0x7fffffffe3b8) at example.c:5
5 if (argc < 2) {
With this setup, GDB will execute the commands specified in gdb_commands.txt
, making your debugging process more organized.
Conclusion
Integrating GDB into your Bash scripts can significantly enhance your debugging workflow. By understanding how to run GDB with command-line arguments, set breakpoints, and automate commands, you can efficiently troubleshoot your applications. This tutorial has provided you with the essential steps to get started with GDB in a Bash script, empowering you to debug more effectively.
FAQ
-
How can I check if GDB is installed?
You can check if GDB is installed by running the command gdb –version in your terminal. -
Can I run GDB on Windows?
Yes, GDB can be run on Windows using MinGW or Cygwin. -
What is the purpose of the -ex option in GDB?
The -ex option allows you to specify commands that GDB will execute upon starting. -
How do I set a breakpoint in GDB?
You can set a breakpoint by using the break command followed by the function name or line number. -
Is it possible to run GDB without a Bash script?
Yes, you can run GDB directly from the command line without using a Bash script.