How to Get User Input in Bash
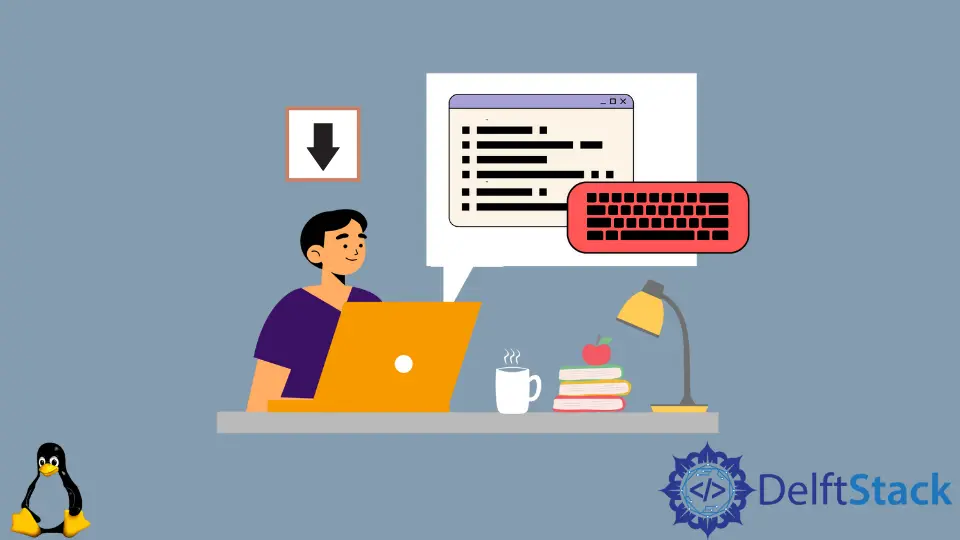
This article will see how to get user input and assign it to a variable in a Bash script.
Use of the read
Command to Get User Input
In Bash, we use the read command to read input from the user.
Example:
echo "Tell me your name: "
read fullname
echo "Hello, " $fullname
Output:
Tell me your name:
JOHN
Hello, JOHN
Above, we echo the user to say their name. Then, read the input from the user and store it in the fullname
variable. Lastly, they are greeted with a Hello
and the name provided by the user.
Use of -p
and -s
Options With the read
Command
We can customize the behavior of the read
command by combining it with other parameters. Some of them include -p
, which allows us to obtain a prompt, and -s
, which silences the input for privacy.
Example:
read -p "Enter username: " username
read -sp "Enter password: " password
echo
echo "Logged in successfully as " $username
Output:
Enter username: johndoe
Enter password:
Logged in successfully as johndoe
In the example above, the script prompts the user to enter a username and stores it in the username
variable. After that, it prompts the user to enter a password but will hide the user’s input and keep it in the password
variable.