How to Read File Lines in Bash
Fumbani Banda
Feb 02, 2024
Bash
Bash File
-
Read File Line by Line in Bash With
read
Command -
Read File Line by Line in Bash With
IFS
and-r
Option
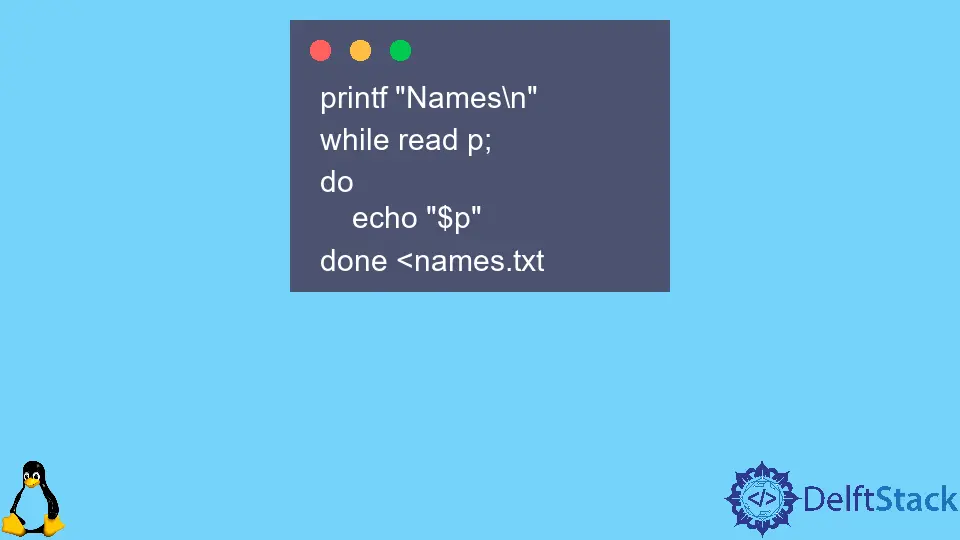
This tutorial reads a file line by line in a bash script with the read
command.
Here is the content of the text file that we will use.
john\n ,
james\n ,
joe,
jack,
jill
Read File Line by Line in Bash With read
Command
names.txt
file is redirected to the while
loop. The read
command processes each line in the file and assigns it to the p
variable, and the echo
command displays it. Once all lines are processed, the while
loop stops. The read
command ignores the leading whitespaces, which can be misleading in some cases.
printf "Names\n"
while read p;
do
echo "$p"
done <names.txt
Output:
Names
johnn ,
jamesn ,
joe,
jack,
jill
Read File Line by Line in Bash With IFS
and -r
Option
By default, the read
command removes all leading whitespaces and interprets the backslash as an escape character. We add the -r
option to disable backslash escaping, and to disable trimming whitespaces, so that the internal field separator(IFS) is cleared.
printf "Names\n"
while IFS= read -r line; do
printf '%s\n' "$line"
done < names.txt
Output:
Names
john\n ,
james\n ,
joe,
jack,
jill
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Fumbani Banda