How to Generate Random Number in Bash
-
Using the
$RANDOM
Variable to Generate Random Numbers -
Using the
shuf
Command to Generate Random Numbers -
Using
/dev/urandom
to Generate Random Numbers - Using Awk to Generate Random Numbers
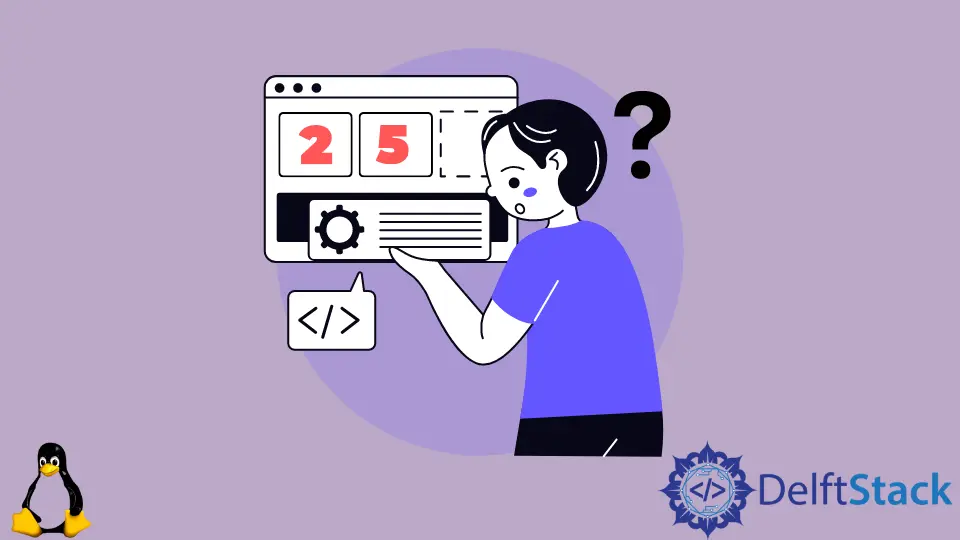
In Bash, an integer or float can be used to produce a random number. A bash script may be used to create a random number within a given range or size. This article demonstrates various methods for generating random numbers in Bash.
Using the $RANDOM
Variable to Generate Random Numbers
The $RANDOM
variable can be used to produce a random number or a range of random values between 0
and 32767
. However, you may limit the number range used to generate random numbers by dividing the $RANDOM
value by a specific value.
To produce a random number between 0 and 32767, use the following command:
$ echo $RANDOM
Output:
28091
You may produce a random number from a specific range by dividing the $RANDOM
variable by the remaining value. It contains double first parentheses (())
with a $
symbol.
To produce a random number between 1 and 100, use the following command:
$ echo $(( $RANDOM % 100 + 1 ))
Output:
19
Since the percentage sign causes the mathematical operation modulo to fail, the number 100 is never attained (remainder after division). If the random generator returned the value 100, the modulo operation would provide the value 0.
Using the shuf
Command to Generate Random Numbers
You may use the command shuf
to produce numerous numbers in a shell script.
The following command will create a single integer between 0 and 100:
$ shuf -i 0-100 -n1
Output:
76
In the above command, -i
denotes the input range, and -n
denotes the headcount. The key-n
is followed by any positive number, indicating the number to generate random numbers. Run the following command to print five random numbers between 0 and 100.
$ shuf -i 0-100 -n5
Output:
68
78
24
76
41
Using /dev/urandom
to Generate Random Numbers
The /dev/urandom
device file allows you to produce pseudo-random numbers far more random than the $RANDOM
variable. However, putting these numbers into variables in a script involves further work, such as filtering via od
, as shown in the example.
od /dev/urandom -A n -t d -N 1
The -t d
option denotes that signed decimal should be used as the output format, and -N 1
tells the program to get one byte from /dev/urandom
.
Output:
124
Using Awk to Generate Random Numbers
The rand()
function of awk
returns a random floating-point number in the range of 0 - 1. The commands that follow BEGIN
will be executed before the next step.
awk 'BEGIN{x=rand(); print x}'
Output:
0.444937