How to Pause Program Execution in Linux Bash
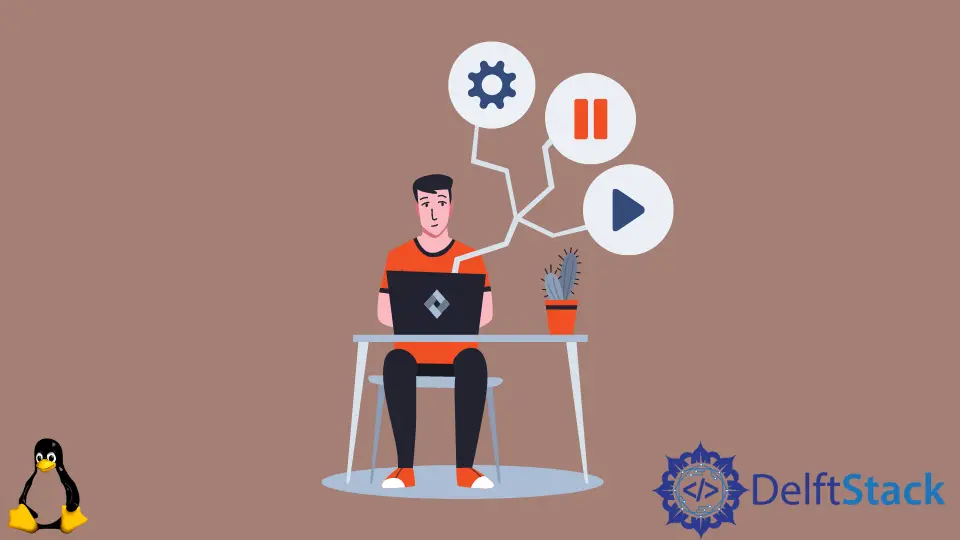
You may sometimes want to pause the program from executing in bash scripting. This could be because of getting input from the user or asking them to confirm a case.
In DOS, you can do this with the pause
command. This article will explain how to pause the program from executing with the read
command.
Pause Program Execution With the read
Command in Bash
The read
command causes the program to pause until an input is received from the user or for a certain amount of time. In the rest of the article, we will explain the parameters of this command and give examples of its different uses.
The following example takes a character from the user to continue executing.
read -n1 -rp "Press any key to continue: " key
- The
-n
parameter specifies the number of characters to be taken as input. In this example, it is set to 1. - The
-r
parameter specifies that the input will be in raw mode. The escape characters are not allowed. - The
-p
parameter creates a prompt. If used with$
and' '
, it allows escaping characters in the text to be printed. It is printed as plain text when used with double-quotes. - The
key
is any variable. It stores the character given as input.
Users can press the Enter key to continue in the example below. They do not have to use a character.
Also, in the example, the $
and ' '
characters are used with the -p
parameter. It allows the \n
character to be used as a newline character.
read -rsp $'Press enter to continue\n'
- The
-s
parameter is used for silent mode. The character taken from the keyboard is not reflected on the screen.
In the next example, the user must press a key within 3 seconds
, or the program will continue to execute.
read -t3 -n1 -rp "Press [x] to exit in 3 seconds " key
- The
-t
parameter specifies a timeout in seconds. In this example, it is set to 3 seconds.
The letter N
is printed to the screen by default in the last example.
read -rp "Do you want to exit? (y/N) : " -ei "N" key;
- The
-e
parameter is used to switch toreadline
mode. - The
-i "N"
parameter specifies the default character forreadline
mode.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn