How to Pass Parameter in a Bash Function
- Understanding Bash Functions
- Passing Parameters to a Bash Function
- Using Multiple Parameters
- Handling Default Values
- Conclusion
- FAQ
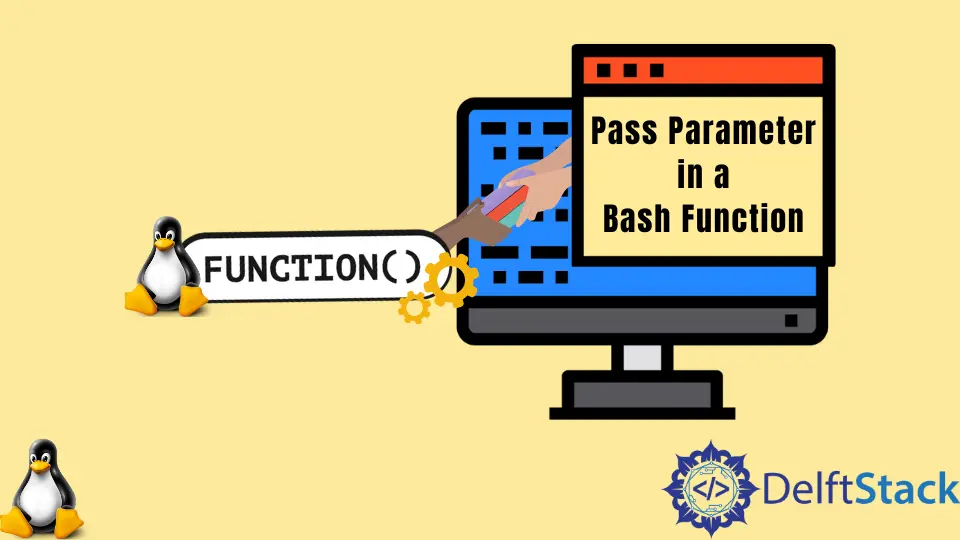
Bash scripting is a powerful tool for automating tasks in Unix-like operating systems. One of the key features that make Bash functions incredibly versatile is the ability to pass parameters.
In this tutorial, we’ll explore how to pass parameters in a Bash function. Whether you’re a seasoned developer or just starting out, understanding how to use parameters can significantly enhance your scripts. By the end of this article, you’ll be equipped with practical examples and insights that will allow you to write more dynamic and flexible Bash scripts. Let’s dive in!
Understanding Bash Functions
Before we get into passing parameters, let’s briefly cover what a Bash function is. A function in Bash is a block of reusable code that can be executed whenever called. It helps in organizing code, making scripts easier to read, and reducing redundancy.
Here’s the basic syntax for defining a function:
function_name() {
# commands
}
You can call the function by simply using its name followed by any parameters you want to pass. Understanding how to pass parameters is crucial, as it allows you to make your functions more dynamic.
Passing Parameters to a Bash Function
In Bash, the parameters passed to a function are accessed using special variables. The first parameter is accessed using $1
, the second with $2
, and so on. You can also use $@
to refer to all parameters passed to the function.
Here’s a simple example to illustrate this:
greet() {
echo "Hello, $1! Welcome to Bash scripting."
}
greet "Alice"
Output:
Hello, Alice! Welcome to Bash scripting.
In this example, the function greet
takes one parameter, which is the name of the person being greeted. When we call greet "Alice"
, the script outputs a personalized greeting. This showcases how passing parameters can make your functions more interactive and user-friendly.
Using Multiple Parameters
You can also pass multiple parameters to your Bash functions. This allows for more complex operations and enhances the functionality of your scripts. Here’s how you can do it:
calculate() {
local sum=$(( $1 + $2 ))
echo "The sum of $1 and $2 is: $sum"
}
calculate 5 10
Output:
The sum of 5 and 10 is: 15
In this example, the calculate
function takes two parameters. We use arithmetic expansion to compute the sum of the two numbers. When we call calculate 5 10
, it outputs the sum, demonstrating the ability to handle multiple parameters effectively.
Handling Default Values
Sometimes, you may want to provide default values for parameters in case none are passed. This can be achieved using conditional statements. Here’s a practical example:
greet() {
local name=${1:-"Guest"}
echo "Hello, $name! Welcome to Bash scripting."
}
greet "Alice"
greet
Output:
Hello, Alice! Welcome to Bash scripting.
Hello, Guest! Welcome to Bash scripting.
In this example, the function greet
uses the syntax ${1:-"Guest"}
to set a default name of “Guest” if no parameter is provided. This ensures that the function can still execute smoothly without any input, making it more robust and user-friendly.
Conclusion
Passing parameters in Bash functions is a fundamental skill that can enhance your scripting capabilities significantly. By understanding how to use positional parameters, handle multiple inputs, and set default values, you can create more dynamic and flexible scripts. Whether you’re automating tasks or building complex applications, mastering these techniques will undoubtedly make your Bash scripting more effective and enjoyable. Happy scripting!
FAQ
-
How do I define a function in Bash?
You can define a function in Bash using the syntaxfunction_name() { # commands }
. -
How can I pass multiple parameters to a Bash function?
You can pass multiple parameters by separating them with spaces when calling the function, and access them using$1
,$2
, etc. -
What if I want to set a default value for a parameter?
You can use the syntax${1:-"default_value"}
to set a default value if no parameter is provided. -
Can I pass parameters of different types to a Bash function?
Yes, Bash functions can accept any type of input, including strings and numbers, as parameters. -
How do I access all parameters passed to a Bash function?
You can use$@
to access all parameters passed to the function.