How to Parse JSON in Bash
-
Use
jq
(Lightweight, Flexible Command-Line JSON Processing Tool) to Parse JSON in Bash -
Use
grep
to Parse JSON in Bash -
Use
python3
to Parse JSON
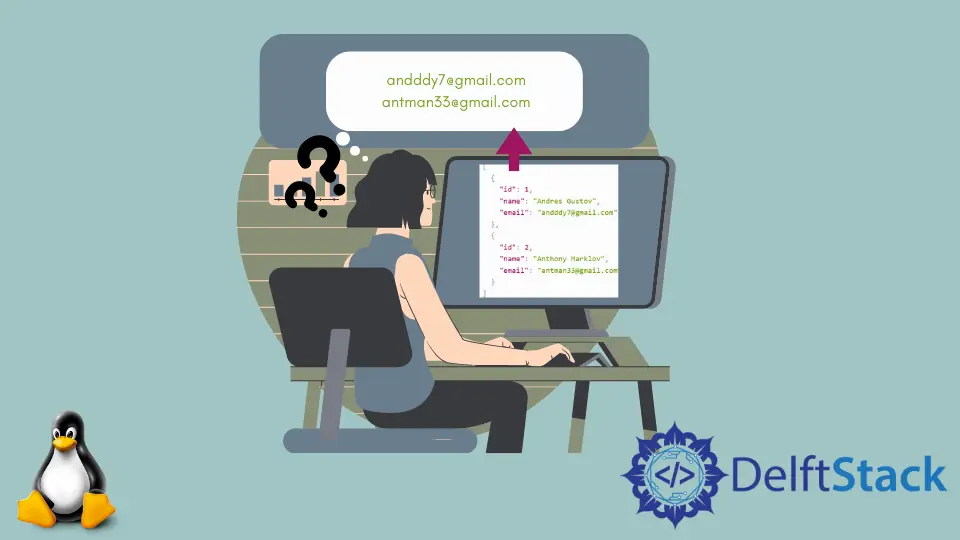
We will learn to parse the JSON data in bash using different techniques.
We will use a fake JSON server for the examples.
Fake JSON server - https://jsonplaceholder.typicode.com/posts
Use jq
(Lightweight, Flexible Command-Line JSON Processing Tool) to Parse JSON in Bash
jq
is a small cross-platform solution for managing JSON data in a shorter, simpler, and effortless way.
You can download jq
from here.
Get Prettier Formatted JSON Data Using jq
jq .
command prettifies the json data.
curl "https://jsonplaceholder.typicode.com/posts" | jq .
Output:
[
{
"userId": 1,
"id": 1,
"title": "delectus aut autem",
"completed": false
},
{
"userId": 1,
"id": 2,
"title": "quis ut nam facilis et officia qui",
"completed": false
},
{
"userId": 1,
"id": 3,
"title": "fugiat veniam minus",
"completed": false
}
... // remaining list of data
]
Get Specific Field’s Values From JSON
We can get values of any specific field from the array of JSON data using jq.[].field_name
.
curl "https://jsonplaceholder.typicode.com/posts" | jq '.[].id'
Output:
1
2
3
...
Get the Title of the First Item From JSON
curl "https://jsonplaceholder.typicode.com/posts" | jq '.[0].title'
Output:
"delectus aut autem"
Use grep
to Parse JSON in Bash
grep
command can also be used for parsing JSON data.
Example JSON file:
[
{
"id": 1,
"name": "Andres Gustov",
"email": "andddy7@gmail.com"
},
{
"id": 2,
"name": "Anthony Marklov",
"email": "antman33@gmail.com"
}
]
Example script:
grep -o '"email": "[^"]*' examplejsonfile.json | grep -o '[^"]*$'
We use the -o
option to select only lines that match the given pattern. Then, we specify the pattern '"email": "[^"]*'
, which means we want all of the values of the key email
. Following that, we pass the JSON file to look for the pattern. Finally, we pipe out the result with another grep -o
command to remove everything except the value.
Output:
andddy7@gmail.com
antman33@gmail.com
Use python3
to Parse JSON
We can also use the json
module of python to handle JSON operations.
curl -s 'https://jsonplaceholder.typicode.com/posts' | \
python3 -c "import sys, json; print(json.load(sys.stdin))"
Get Specific Field Value
curl "https://jsonplaceholder.typicode.com/posts" | \
python3 -c "import sys, json; data=json.load(sys.stdin); print([d['id'] for d in data])"
Output:
1
2
3
...
Get the Title of the First Item
curl "https://jsonplaceholder.typicode.com/posts" | \
python3 -c "import sys, json; print(json.load(sys.stdin)[0]['title'])"
Output:
"delectus aut autem"