How to Parse Command Line Arguments in Bash
- Parsing Positional Parameters in Bash
- Parsing Arguments With Flags in Bash
- Parsing Arguments With a Loop Construct in Bash
-
Parsing Arguments With
shift
in Bash
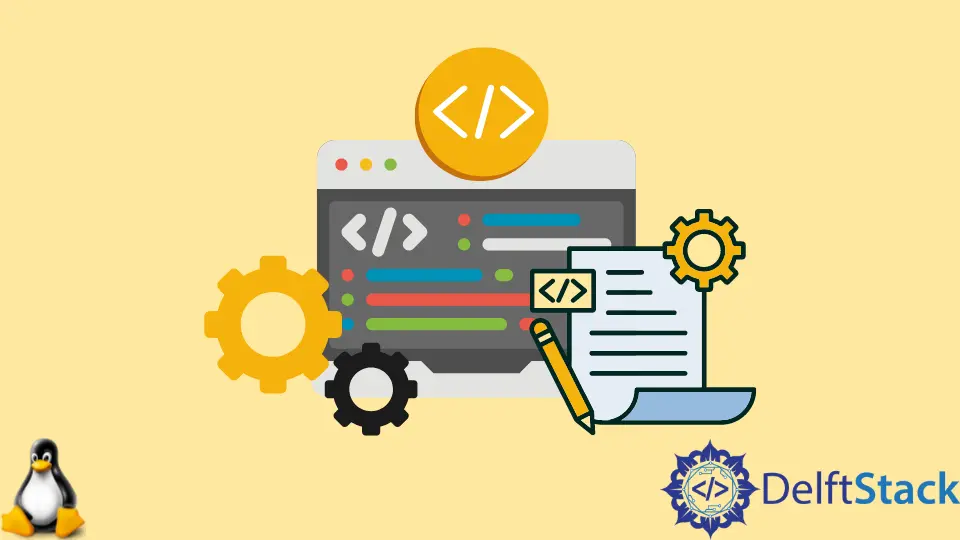
This tutorial demonstrates parsing command-line arguments to bash script as positional parameters, using flags, loop construct, and shift operator.
Parsing Positional Parameters in Bash
Positional parameters are accessed in the order that they are passed to the bash script. The first parameter is accessed by $1
, the second by $2
, and so on.
echo "I am $1";
echo "And I live in $2";
Run the script with two positional arguments:
bash positional_args.sh John USA
Output:
I am John
And I live in USA
Parsing Arguments With Flags in Bash
A flag begins with a hyphen -
before each argument. The order of the arguments does not matter when flags are used.
In the script below, getopts
reads the flags from the input and OPTARG
matches it to the corresponding value.
while getopts n:c: flag
do
case "${flag}" in
n) name=${OPTARG};;
c) country=${OPTARG}
esac
done
echo "I am $name";
echo "And I live in $country";
Run:
bash flags.sh -n fumbani -c Malawi
Output:
I am fumbani
And I live in Malawi
Parsing Arguments With a Loop Construct in Bash
The loop construct is useful when the argument size is not known in advance. The $@
is a variable containing all the input parameters. The for
loop iterates over all the arguments and processes each argument passed.
i=1
for arg in "$@"
do
printf "argument $i: $arg\n"
i=$((i + 1 ))
done
Run:
bash arguments_loop.sh USA Europe Africa Asia
Output:
argument 1: USA
argument 2: Europe
argument 3: Africa
argument 4: Asia
Parsing Arguments With shift
in Bash
The shift
operator makes the indexing of the arguments to start from the shifted position. In our case, we are shifting the arguments by 1
until we reach the end.$#
refers to the input size, and $1
refers to the next argument each time.
i=1
max=$#
while (( $i <= $max ))
do
printf "Argument $i: $1\n"
i=$((i + 1 ))
shift 1
done
Run:
bash shift.sh one two three
Output:
Argument 1: one
Argument 2: two
Argument 3: three