How to Modify a Global Variable Within a Function in Bash
- Understanding Variable Scope in Bash
- Method 1: Direct Assignment
-
Method 2: Using the
declare
Command -
Method 3: Using the
eval
Command - Conclusion
- FAQ
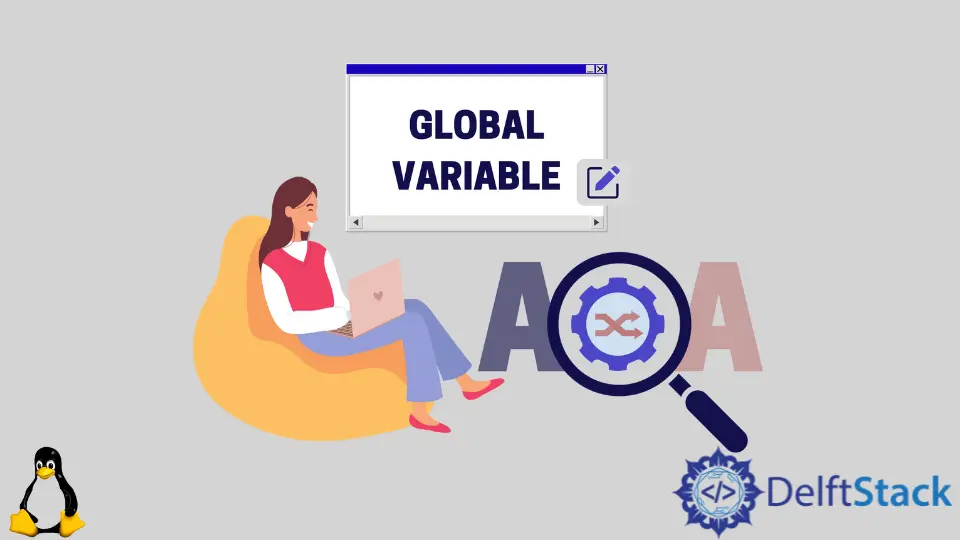
When working with Bash scripting, understanding how to manipulate global variables within functions can be a game changer. Whether you’re writing a simple script or a complex automation tool, being able to modify a global variable allows for greater flexibility and control over your program’s behavior.
In this tutorial, we will explore various methods to modify a global variable within a function in Bash. We’ll cover the nuances of variable scope and demonstrate practical examples to help you grasp these concepts easily. So, if you’re ready to enhance your Bash scripting skills, let’s dive in!
Understanding Variable Scope in Bash
Before we jump into the methods of modifying global variables, it’s crucial to understand how variable scope works in Bash. In Bash, variables defined outside of any function are considered global. Conversely, variables defined within a function are local to that function by default. This distinction is important because it dictates how you can access and modify variables throughout your script.
To modify a global variable from within a function, you can simply reference the variable by its name. However, if you want to ensure that the variable is modified correctly, you may need to use specific techniques. Let’s explore these methods in detail.
Method 1: Direct Assignment
The simplest way to modify a global variable within a function is through direct assignment. This method involves referencing the global variable directly and assigning a new value to it. Here’s how it works:
#!/bin/bash
global_var="Initial Value"
modify_global() {
global_var="Modified Value"
}
modify_global
echo $global_var
Output:
Modified Value
In this example, we start with a global variable global_var
initialized to “Initial Value”. The function modify_global
directly modifies this variable by assigning it a new value, “Modified Value”. After calling the function, when we echo the variable, we see that the change has taken effect. This method is straightforward and effective for simple scripts where variable scope is not a concern.
Method 2: Using the declare
Command
Another method to modify a global variable within a function is by using the declare
command. This command allows you to explicitly define the variable as global, ensuring that any modifications you make within the function persist outside of it. Here’s an example:
#!/bin/bash
global_var="Initial Value"
modify_global() {
declare -g global_var="Modified Value"
}
modify_global
echo $global_var
Output:
Modified Value
In this script, we again start with the global variable global_var
. Inside the modify_global
function, we use the declare -g
command to indicate that we are modifying the global variable. This ensures that the new value, “Modified Value”, is accessible outside the function. When we echo global_var
after calling the function, we confirm that the variable has been successfully modified. This method is particularly useful when you want to avoid accidental shadowing of global variables.
Method 3: Using the eval
Command
If you want to modify a global variable using a more dynamic approach, you can utilize the eval
command. This method allows you to construct a command as a string and then execute it, which can be particularly useful in more complex scripts. Here’s how it works:
#!/bin/bash
global_var="Initial Value"
modify_global() {
local new_value="Modified Value"
eval "global_var=\"$new_value\""
}
modify_global
echo $global_var
Output:
Modified Value
In this example, we define global_var
as before. Inside the modify_global
function, we create a local variable new_value
and use eval
to construct a command that modifies global_var
. By executing this command, we effectively change the value of the global variable. This method is powerful, but it should be used with caution, as it can introduce complexity and potential security risks if not handled properly.
Conclusion
Modifying global variables within functions in Bash is a fundamental skill that can significantly enhance your scripting capabilities. Whether you choose direct assignment, the declare
command, or the eval
command, understanding these methods will empower you to write more dynamic and effective scripts. As you practice these techniques, you’ll find that managing variable scope becomes second nature, allowing you to create more robust Bash scripts. Happy scripting!
FAQ
-
how do global and local variables differ in Bash?
Global variables can be accessed from anywhere in the script, while local variables are confined to the function in which they are defined. -
can I declare a variable as global inside a function?
Yes, you can use thedeclare -g
command to declare a variable as global within a function. -
what is the purpose of the eval command in Bash?
Theeval
command allows you to construct and execute commands dynamically, which can be useful for modifying variables in complex scenarios. -
are there any risks associated with using eval?
Yes, usingeval
can introduce security risks, especially if you’re executing commands constructed from user input. Always validate and sanitize inputs. -
how can I check the value of a global variable after modifying it?
You can simply use theecho
command to print the value of the global variable after it has been modified within the function.