How to Loop Over Files in Directory in Bash
- Loop Through Files
- Loop Over Directory
- Write All Files Inside the Directory Using Loop
- Make Backups of All Files Inside the Directory
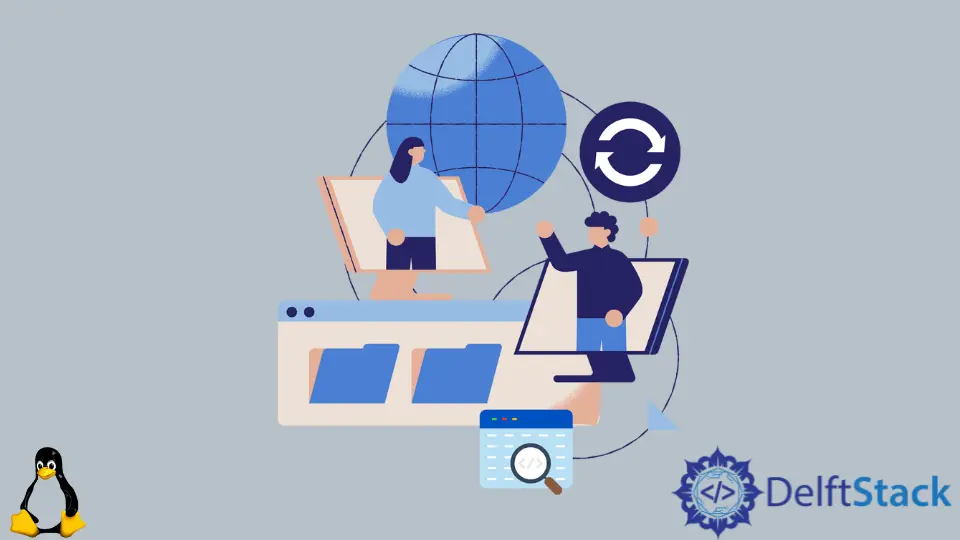
Loops in Bash have made it feasible to do operations on many files. You must have some privileges to work with files and folders.
The most effective method is looping, which allows the user to apply the same logic to the object again by utilizing a simple code line.
Loop Through Files
First, we will create a test
directory and create multiple files inside the directory. Let’s create five files in test
directory as file1.txt
, file2.txt
, file3.txt
, file4.txt
, and file5.txt
.
We created a test
folder using mkdir
and created five files inside it using the touch
command.
Loop Over Directory
Let’s loop over the newly created test
directory and display the file names inside the directory. We’ll use for
loop to do this.
~/test$ for file in *; do echo $file; done
Navigate to the test
directory and enter the above command after $
. *
denotes all files inside the directory.
Output:
file1.txt
file2.txt
file3.txt
file4.txt
file5.txt
Write All Files Inside the Directory Using Loop
To write Hello World!
in each file, we’ll use the for
loop to iterate over files and the -e
flag in echo
to preserve newlines.
~/test$ for file in *; do echo -e "$file\nHello World!"> $file ; done
$file
will show the file name at the beginning of the file, and \n
will break the line, and Hello World!
will be previewed in the second line. To check the data we added to the file, we need to display it using cat
with a for
loop.
~/test$ for file in *; do cat $file ; done
Output:
file1.txt
Hello World!
file2.txt
Hello World!
file3.txt
Hello World!
file4.txt
Hello World!
file5.txt
Hello World!
Make Backups of All Files Inside the Directory
.bak
extension denotes the backup files. We’ll use cp
with for
loop to create backups.
~/test$ for file in *; do cp $file "$file.bak" ; done
You can use ls -l
to list all the files inside the test
directory.
~/test$ ls -l
Let’s check out the test
directory.
You can see that backup files were created with suffixed .bak
extension inside the folder.