How to Use the sleep Command in Bash
-
Understanding the
sleep
Command -
Basic Usage of the
sleep
Command - Using Sleep in Conditional Statements
- Combining Sleep with Other Commands
- Tips for Using Sleep Effectively
- Conclusion
- FAQ
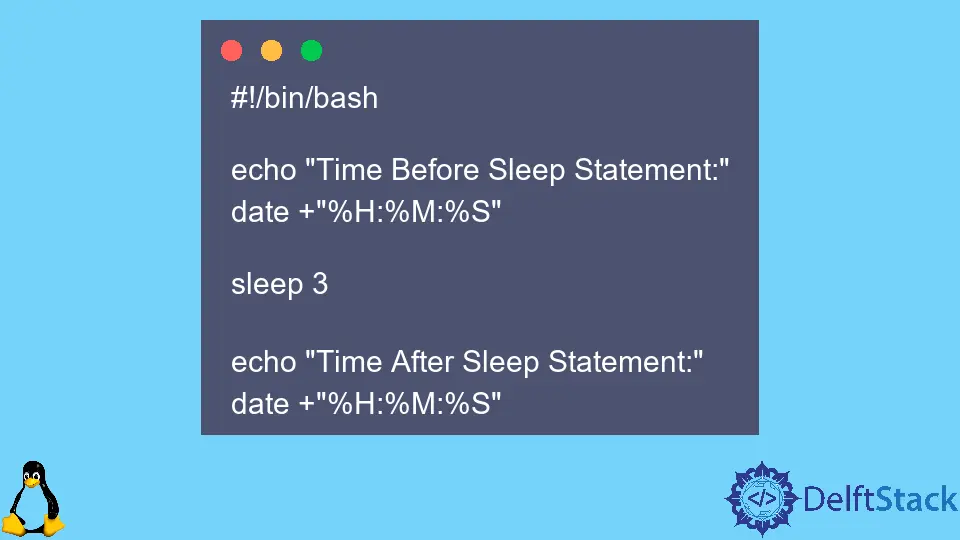
The sleep command in Bash is a simple yet powerful tool that allows you to pause the execution of your scripts or commands for a specified period. Whether you’re automating tasks, managing system processes, or simply need to introduce a delay, understanding how to use the sleep command effectively can enhance your Bash scripting skills.
In this article, we will explore various ways to implement the sleep command in Bash, complete with illustrative code examples. By the end, you’ll have a solid grasp of how to use this command to control the timing of your scripts, making them more efficient and user-friendly.
Understanding the sleep
Command
The sleep command is straightforward: it halts the execution of the following command for a specified duration. This duration can be expressed in seconds, minutes, hours, or even days, depending on your needs. The syntax is simple:
sleep [NUMBER][SUFFIX]
Here, NUMBER is the amount of time you want to pause, and SUFFIX is optional, where you can specify ’s’ for seconds, ’m’ for minutes, ‘h’ for hours, and ’d’ for days. If no suffix is provided, the default is seconds.
Using sleep effectively can be particularly useful in scripts that require waiting for certain processes to finish or when you want to avoid overwhelming system resources by executing commands too quickly.
Basic Usage of the sleep
Command
To start, let’s look at a simple example of how to use the sleep command in a Bash script.
#!/bin/bash
echo "Starting the process..."
sleep 5
echo "Process resumed after 5 seconds."
Output:
Starting the process...
Process resumed after 5 seconds.
In this script, the first echo command prints a message indicating the start of a process. Then, the sleep command pauses execution for 5 seconds before the second echo command runs, which informs the user that the process has resumed. This is particularly useful when you want to give users a moment to read the initial output before moving on.
The sleep command can also be used in loops. For example, if you want to create a simple countdown timer, you could do something like this:
#!/bin/bash
for i in {5..1}; do
echo "$i..."
sleep 1
done
echo "Time's up!"
Output:
5...
4...
3...
2...
1...
Time's up!
This script counts down from 5 to 1, pausing for one second between each number. After the countdown, it announces that the time is up. This illustrates how sleep can be integrated into loops to create timed events or delays.
Using Sleep in Conditional Statements
Another powerful way to use the sleep command is within conditional statements. This allows you to introduce delays based on specific conditions, enhancing the interactivity of your scripts.
#!/bin/bash
read -p "Do you want to proceed? (yes/no): " answer
if [ "$answer" == "yes" ]; then
echo "Proceeding..."
sleep 2
else
echo "Exiting..."
sleep 1
fi
Output:
Do you want to proceed? (yes/no): yes
Proceeding...
In this example, the script prompts the user for input. If the user responds with “yes,” it pauses for two seconds before proceeding. If the answer is anything else, it pauses for one second before exiting. This use of sleep within conditional statements makes the script more user-friendly and interactive.
Combining Sleep with Other Commands
The sleep command can also be combined with other commands to create more complex scripts. For instance, you might want to run a command, wait for a moment, and then execute another command based on the outcome of the first.
#!/bin/bash
echo "Updating package lists..."
sudo apt update
sleep 3
echo "Installation complete."
Output:
Updating package lists...
Installation complete.
In this script, the sleep command is used after the package update command. This way, users can see the message indicating the update is in progress before it moves on to the next step. It provides a clearer flow of information, especially in longer scripts where multiple commands are executed in sequence.
Tips for Using Sleep Effectively
While the sleep command is simple, there are some best practices to keep in mind for effective usage:
- Keep it Short: Use the sleep command judiciously. Long pauses can lead to frustration for users waiting for scripts to complete.
- Use Descriptive Messages: Always inform users why a pause is occurring, especially if it’s for a significant duration.
- Test Your Scripts: Before deploying scripts with sleep commands, test them to ensure the timing feels right and the flow is logical.
Incorporating these tips will help you create more efficient and user-friendly scripts.
Conclusion
The sleep command in Bash is a versatile tool that can significantly improve the functionality of your scripts. By adding pauses, you can control the flow of execution, create interactive prompts, and enhance user experience. Whether you’re a beginner or an experienced scripter, mastering the sleep command will undoubtedly add value to your Bash scripting toolkit. With the examples and explanations provided in this article, you should now feel confident in utilizing sleep in your own scripts to manage timing effectively.
FAQ
-
What is the primary function of the sleep command in Bash?
The sleep command pauses the execution of the next command for a specified amount of time. -
Can I specify the duration in units other than seconds?
Yes, you can specify the duration in minutes (m), hours (h), or days (d) as well.
-
How can I use sleep in a loop?
You can use sleep within a loop to create timed events, such as countdowns. -
Is it possible to use sleep in conditional statements?
Absolutely! You can introduce delays based on user input or other conditions. -
What are some best practices for using the sleep command?
Use short pauses, provide descriptive messages, and test your scripts for logical flow.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn