How to Concatenate Strings in Bash
- Method 1: Basic Concatenation
- Method 2: Using the += Operator
- Method 3: Concatenating Multiple Strings
- Method 4: String Concatenation in Loops
- Method 5: Using Command Substitution
- Conclusion
- FAQ
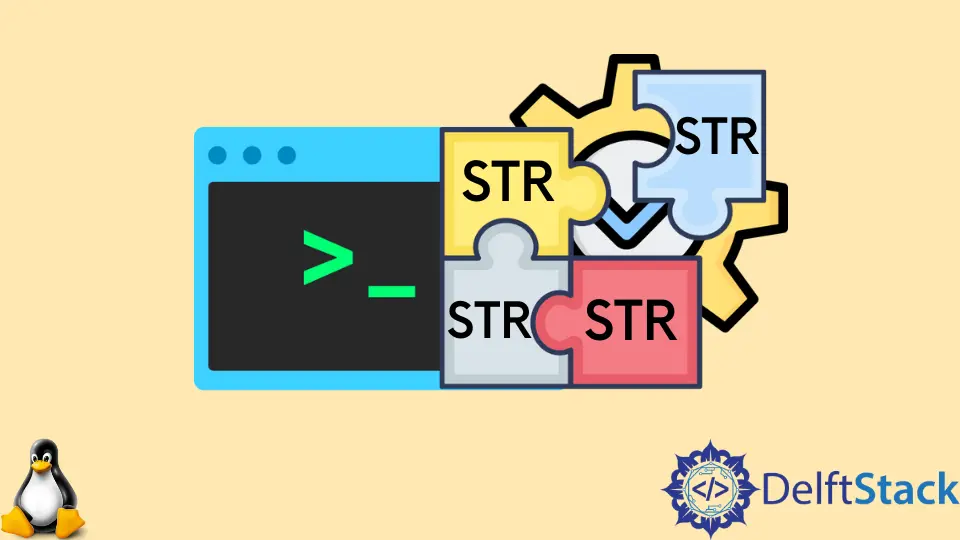
Concatenating strings in Bash might seem like a daunting task for beginners, but it’s actually quite straightforward. Whether you’re working with simple variables or complex scripts, knowing how to combine strings can significantly enhance your scripting capabilities. In Bash, you can concatenate two or more string variables by simply placing them next to each other or by using the +=
operator.
This article will walk you through various methods to concatenate strings in Bash, providing clear examples and explanations to help you master this essential skill.
Method 1: Basic Concatenation
The simplest way to concatenate strings in Bash is to write them one after the other. This method is intuitive and works seamlessly for most cases. Let’s take a look at a basic example.
string1="Hello"
string2="World"
result="$string1 $string2"
echo $result
Output:
Hello World
In this example, we define two string variables, string1
and string2
. By placing them next to each other within double quotes, we create a new variable called result
. The space between the two strings is added by including a space in the quotes. When we echo the result
, it displays “Hello World”. This method is particularly useful for combining static strings or variables without any additional formatting.
Method 2: Using the += Operator
Another effective way to concatenate strings in Bash is through the +=
operator. This operator allows you to append a string to an existing variable, making it very handy when dealing with loops or conditional statements.
greeting="Hello"
greeting+=" World"
echo $greeting
Output:
Hello World
In this example, we start with a variable named greeting
initialized to “Hello”. By using the +=
operator, we append " World" to it. The result remains stored in the same variable, allowing for efficient updates. This method is particularly useful when you want to build a string incrementally, such as in a loop where you might be adding multiple strings together.
Method 3: Concatenating Multiple Strings
You can also concatenate multiple strings at once in Bash. This is useful for combining several variables or static strings into one cohesive output.
first="Bash"
second="is"
third="great!"
combined="$first $second $third"
echo $combined
Output:
Bash is great!
In this example, we define three variables: first
, second
, and third
. By placing them together in double quotes and separating them with spaces, we create a new variable called combined
. This method is effective for combining multiple strings into a single output, making it easier to manage larger text outputs or messages.
Method 4: String Concatenation in Loops
When working with loops, concatenating strings can be done dynamically. This method is particularly useful when you need to build a string based on user input or data processed in a loop.
result=""
for word in "Learning" "Bash" "is" "fun"
do
result+="$word "
done
echo $result
Output:
Learning Bash is fun
In this example, we initialize an empty string called result
. The loop iterates through a list of words, appending each word to the result
variable followed by a space. This method is powerful because it allows for dynamic string construction based on varying input, making it ideal for processing lists or arrays.
Method 5: Using Command Substitution
You can also concatenate strings by using command substitution. This technique allows you to incorporate the output of one command into another string.
name="John"
greeting="Hello, $(echo $name)!"
echo $greeting
Output:
Hello, John!
In this example, we use command substitution with $(...)
to include the output of the echo $name
command within the greeting
string. This method is particularly useful when you need to incorporate dynamic data or command results into your strings.
Conclusion
Concatenating strings in Bash is a fundamental skill that can greatly enhance your scripting efficiency. Whether you use basic concatenation, the +=
operator, or more advanced techniques like loops and command substitution, mastering these methods will empower you to create dynamic and engaging scripts. As you continue to explore Bash scripting, remember that the ability to manipulate strings is crucial for effective data handling and user interaction.
FAQ
-
How do I concatenate two strings in Bash?
You can concatenate two strings in Bash by placing them next to each other or using the+=
operator. -
Can I concatenate strings in a loop?
Yes, you can concatenate strings in a loop by appending each string to a result variable. -
What is command substitution in Bash?
Command substitution allows you to use the output of a command as part of a string by enclosing it in$(...)
. -
Is there a limit to string length in Bash?
While there isn’t a strict limit, extremely long strings may cause performance issues or exceed system memory limits. -
Can I concatenate strings with special characters?
Yes, you can concatenate strings with special characters, but be mindful of how they are interpreted by the shell.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn