Hash Tables in Bash
-
Declaring and Adding
<key,value>
Pairs in Dictionary Variable in Bash -
Retrieving
<key,value>
Pairs From the Dictionary in Bash -
Updating
<key,value>
in a Dictionary in Bash -
Removing a
<key,value>
Pair From the Dictionary - Iterating a Dictionary in Bash in Bash
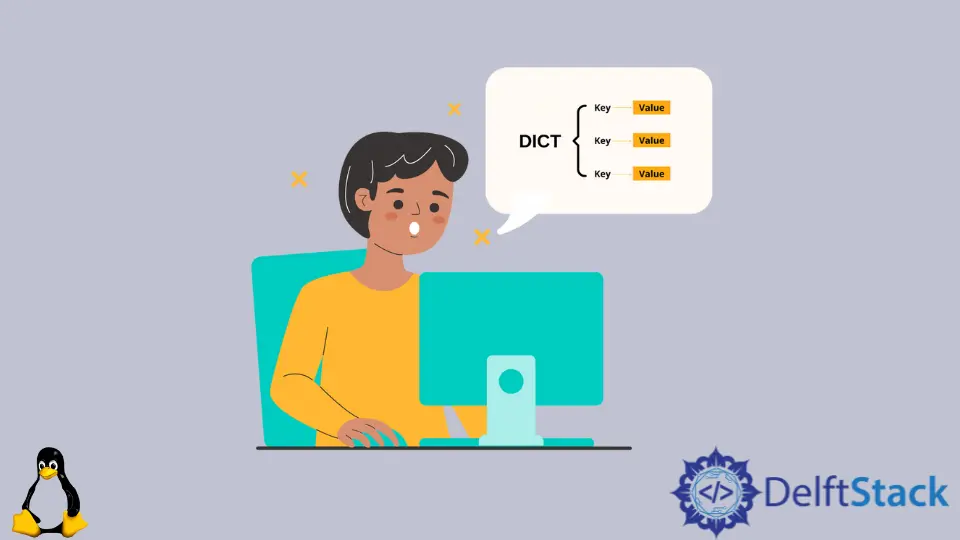
A dictionary, also called a hashmap, or associative array, is one of the most fundamental data structures in any programming language to store a collection of things.
A dictionary is a collection of keys, each of which has a value associated with it. You can insert, retrieve, or change a value in a dictionary using the matching key.
Although Bash is not a general-purpose programming language, it natively supports hashmaps
in versions four and higher.
Declaring and Adding <key,value>
Pairs in Dictionary Variable in Bash
Variables in Bash are not strongly typed, so you must impose type-like behavior by stating a variable’s attribute
.
To utilize a dictionary in Bash, use the declare
statement with the -A
option which means associative array, to declare a dictionary variable.
$ declare -A dict
We’ve now declared a variable called dict
, which can be used like a dictionary. To add <key,value>
pairs to the dictionary, we’ll follow the following syntax:
$ name_of_dictionary[key]=value
Let’s add <key, value>
pairs using the syntax above.
$ dict[1]=Nil
$ dict[2]=Esh
We have added two <key, value>
pairs where keys are 1
and 2
and values are Nil
and Esh
.
Retrieving <key,value>
Pairs From the Dictionary in Bash
To check corresponding values
in the dictionary with a key
, we have to add $
with braces {}
to the dictionary variable we defined.
$ echo ${dict[1]}
Output:
Nil
Updating <key,value>
in a Dictionary in Bash
To update an existing <key, value>
in the dictionary, we must insert a new <key, value>
and overwrite the existing value.
$ dict[1]=Nilesh
If we retrieve the value of key 1
, then:
$ echo ${dict[1]}
Output:
Nilesh
As we can see, Nil
of key 1
is overwritten by Nilesh
.
Removing a <key,value>
Pair From the Dictionary
We use the unset
command to remove a <key, value>
pair.
$ unset dict[1]
The command above will remove the <key, value>
pair with key 1
.
Iterating a Dictionary in Bash in Bash
In Bash, we can use a for
loop to iterate a dictionary. Let’s look at an example:
#!/bin/bash
declare -A dict
dict[1]=Nil
dict[2]=Esh
for key in "${!dict[@]}"; do
echo "$key ${dict[$key]}"
done
We rewrote the preceding command in a script and ran it in a Bash terminal. ""
is used to handle the keys with spaces.
Output: