How to Format Date and Time in Bash
-
Using the Inbuilt
printf
to Format Date and Time in Bash -
Using the
date
Command Format Date and Time in Bash
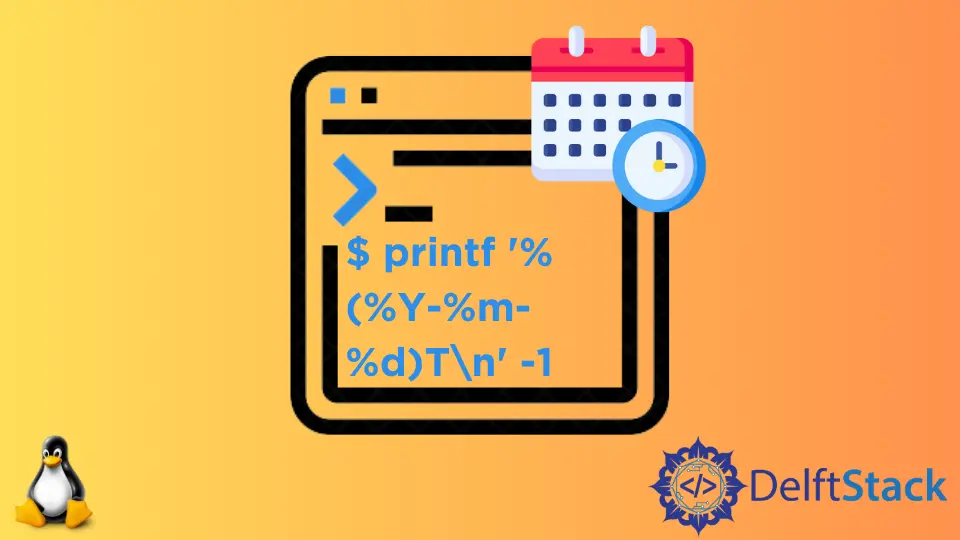
To format a date or time in a Linux shell script, use the standard date command.
The same command can be used with the shell script. The date command in Linux shows and changes the system date and time.
Users may also use this command to print the time in various formats and compute future and previous dates.
Using the Inbuilt printf
to Format Date and Time in Bash
It is better to use the printf
built-in date formatter instead of the external date formatter in a bash version greater than or equal to 4.2
.
To get in YYYY-MM-DD
format:
$ printf '%(%Y-%m-%d)T\n' -1
-1
denotes current time.
Output:
2021-12-21
We can also write the command above as:
$ printf '%(%F)T\n' -1
Output:
2021-12-21
We can save the current time by using -v
and specifying the variable name. To save the current time in a variable cdate
and print it, we can do the following:
$ printf -v cdate '%(%F)T' -1
$ echo $cdate
The above command captured the current date as a variable and stored it in cdate
, and echo
will print the variable’s value.
Output:
2021-12-21
Using the date
Command Format Date and Time in Bash
We can use the date
command for bash versions less than 4.2
.
To display the current date in MM-DD-YY
format:
$ date +"%m-%d-%y"
The +
symbol indicates a user-defined format string that specifies how the date and time should be displayed.
Output:
12-21-21
To convert the year into a four-digit, change y
to Y
.
$ date +"%m-%d-%Y"
Output:
12-21-2021
We can also do the following to print the current date in YYYY-MM-DD
format.
$ date -I
Output:
2021-12-21
To display time only:
$ date +"%T"
Output:
13:01:00
To display time in HH:MM
format:
$ date +"%H-%M"
Output:
13-02
To store the current date in shell variable we can do following:
$ cdate=$(date +"%m-%d-%Y")
$ echo $cdate
We have captured the current date and stored it in cdate
. echo
prints the cdate
value.
Output:
12-21-2021
To add N
days in the date
, we use the following command:
date -d="YYYY-MM-DD+N days"
Let’s look at an example.
$ date -d "2021-12-21+20 days"
Output:
Mon 10 Jan 2022 12:00:00 AM