Fork in Bash
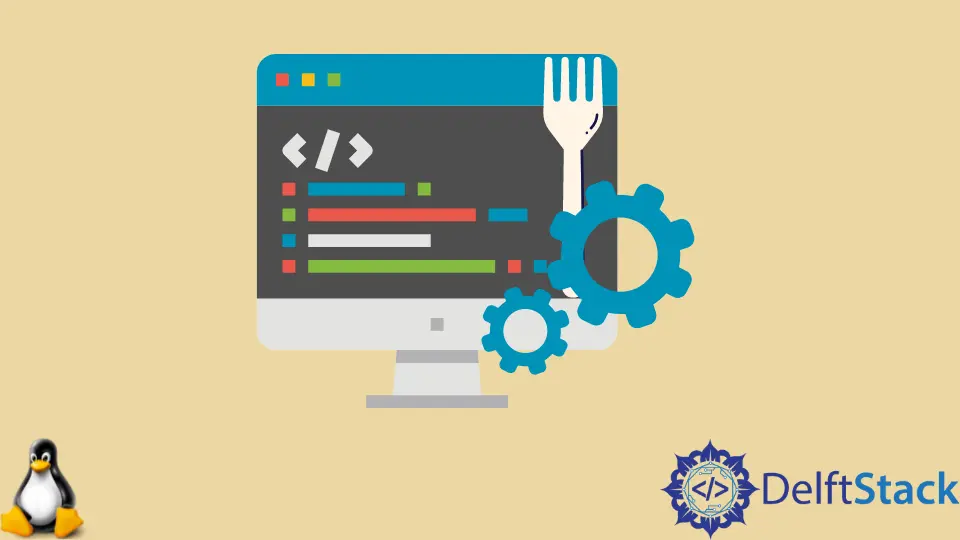
This article presents an introductory tutorial on a Bash equivalent (i.e., subshells) for C/C++ fork
system call for forking new asynchronous child processes.
Fork in Bash
The system call in C/C++ is to create a new process, i.e., the child process of the calling process. After the creation of the new process, both processes will execute asynchronously.
Both have their own address space. None of the processes will wait for the other process to end before its execution ends; however, in Bash, these child processes are known as subshells.
These subshells run in parallel, allowing multiple tasks to be done simultaneously. Therefore, the fork
functionality in Bash can be achieved by creating new subshells.
To create a subshell, we follow the below syntax:
(sub_process) &
We enclose the function or any lines of code to be executed as a subprocess in parenthesis and then put an ampersand &
sign. All the statements inside these parentheses will make a sub-process and be executed as a subshell.
Consider the following example:
In this example, we have created the function child_process()
and wrote some statements. Later, we called that function by enclosing it in parenthesis and placing an &
at the end.
By this, the function will be called in a subshell. The Parent shell will execute its next statements concurrently.
We can see from the output that the parent process (as it was running concurrently) printed its next statement before the child could approach its print
statement.
Variables in Subshells
As far as the variables are concerned, all the shells, whether parent shell or subshell, will have their variables. Since both have their own address space, the process will eventually have separate local variables.
Let us look at the example below:
In this script, we created a variable local_var
in the parent process and set its value. Later in the child_process
function, we assigned it a new value for the subshell.
After creating a child process, the parent process prints the value of the variable, so the parent’s value will be printed.
The output will be as follows:
We can see from the output above that the local variables are not accessible outside the block of code of the child process. Even the parent process cannot access the value set by the child process.