Bash fi Keyword
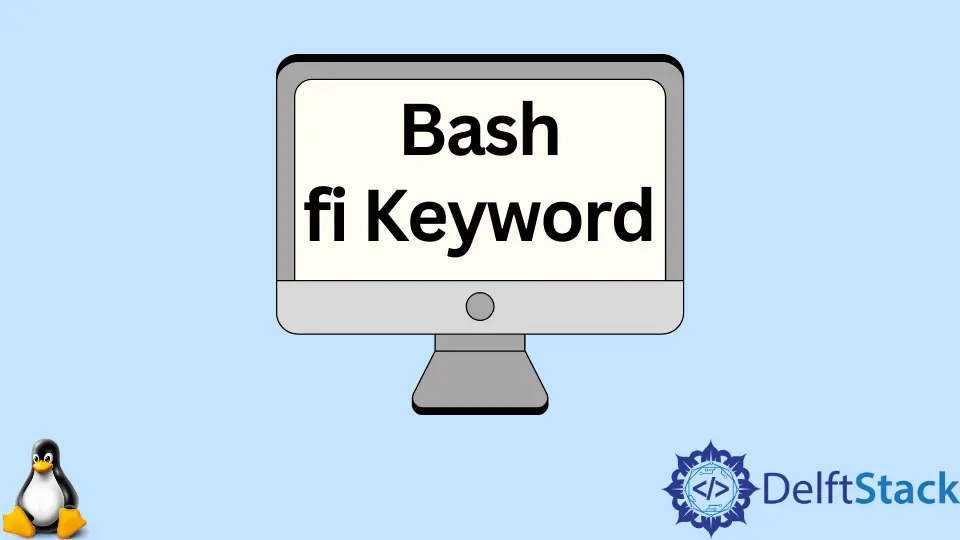
Bash is a scripting language that is specially built for Linux Shell. This is also called shell scripting.
The general format for the if ... else
conditional statement is:
if CONDITIONAL COMMANDS
then
STATEMENTS
fi
In this article, we will see the use of the fi
keyword in Bash. Also, we will see necessary examples to make our topic easier.
the fi
Keyword in Bash
We use the fi
keyword with the if ... else
conditional command. It’s used for indicating that the conditional statements end here.
But it’s very important to include the then
keyword before the fi
keyword; otherwise, it will cause an error in your code. Below shared an example with line by line explanation.
echo -n "Please enter a number: "
read YOUR_VAR
if [[ $YOUR_VAR -gt 10 ]]
then
echo "The input you provided is greater than 10."
fi
Let’s explain the code line by line to make it easier.
- We take the user input to the system through the line
echo -n "Please enter a number: "
. - The system reads the user input through the line
read YOUR_VAR
. - Through the line,
if [[ $YOUR_VAR -gt 10 ]]
, the system checks whether the user input is greater than10
. - Through the keyword
then
, the conditional statement starts, and if the condition matches the criteria, then it will printThe input you provided is greater than 10.
through the lineecho "The input you provided is greater than 10."
. - Lastly, we end the
if ... else
with the keywordfi
.
Please note that the keyword -gt
means Greater Than
. After executing the program on your shell, you will get the output below.
Please enter a number: 12
The input you provided is greater than 10.
From the above example, we can say that all the statements we want to be done by the if ... else
needs to include between the then
and fi
. The keyword then
indicates the start, and the keyword fi
indicates the finish.
So the keyword fi
is always used to end the conditional block.
The major difference between the fi
and ;;
is that the ;;
closes all the current entries of the case
statement, and the fi
closes the if
statement. Both of them work on a conditional statement.
Please note that all the code used in this article is written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn