How to Extract File Base Name in Bash
- Using Substring Parameter Expansion to Extract File Base Name in Bash
-
Using the
basename
Command to Extract File Base Name in Bash
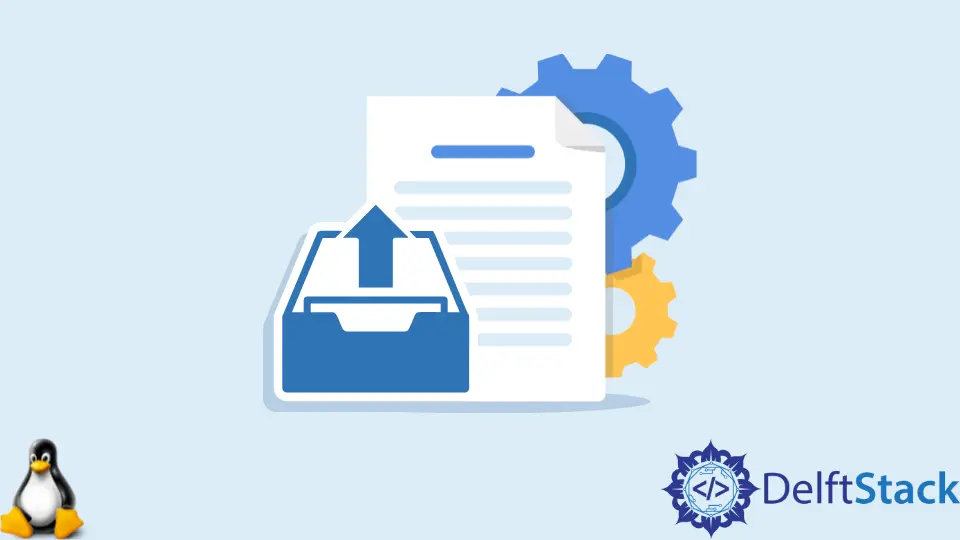
This tutorial shows different ways to extract the file base name using substring parameter expansion and the basename
command.
Using Substring Parameter Expansion to Extract File Base Name in Bash
Parameters in Bash are elements that store values. Expansion is substituting a parameter reference with its value.
To do parameter expansion in Bash, precede the parameter’s name with a $
character. Enclosing the parameter’s name in curly braces is optional.
The two notations for parameter expansion are shown below.
$parameter
${parameter}
Inside the curly braces of parameter expansion, some operators can be added together with their arguments. The operators are placed after the parameter’s name and before the closing curly brace.
We can use these operators for conditional, substring, subset, prefix listing, element counting, and case modification expansion. These operators only modify the parameter without modifying the parameter’s value.
The script below uses substring parameter expansion to extract a substring by string pattern matching. The syntax used is ${parameter##pattern}
.
The ##
specifies that the pattern should match from the string’s left side and the */
is the pattern in our case. The ${path##*/}
matches for the last /
from the path
variable and returns the remaining substring, file1.txt
, in this case.
path=/home/delftstack/test/file1.txt
echo "${path##*/}"
Executing the script prints the name of the file to the standard output.
file1.txt
Using the basename
Command to Extract File Base Name in Bash
The basename
is a command in Linux that returns the last element given a file path as the argument. In the script below, the basename
command is given the path
variable that has the file path as the argument. The script executes the basename
command using command substitution.
Command substitution is a Bash feature that allows us to run Linux commands and store the command’s output in a variable. Once a command is executed using this feature, the command’s standard output replaces the command, with any trailing newlines removed.
The output of the basename
command replaces the command itself and is assigned to the fname
variable. The printf
command is used to display the contents of the fname
variable to the standard output.
path=/home/delftstack/test/file1.txt
fname=$(basename "$path")
printf $fname
printf "\n"
The script displays the following output after it is executed.
file1.txt