How to Handle Errors in Bash
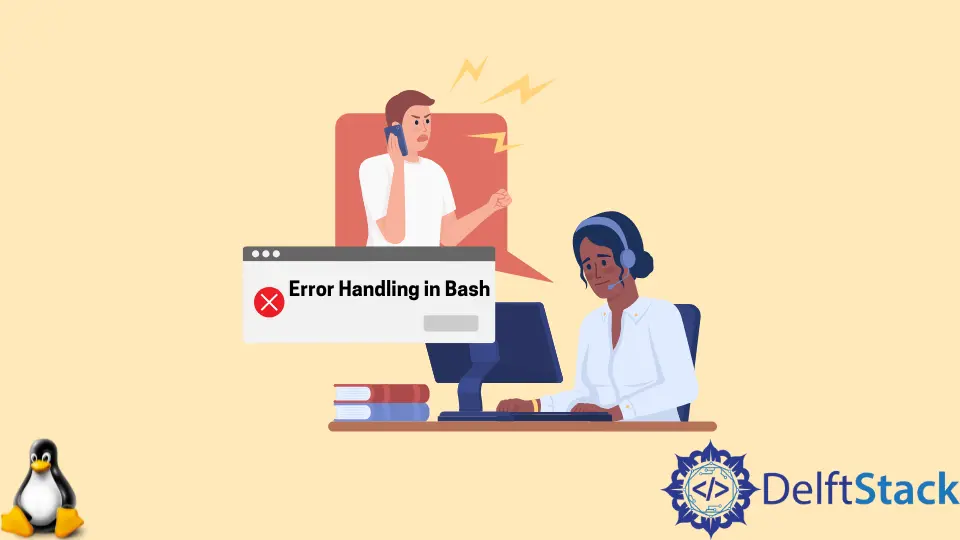
This article teaches error handling in bash. Remember, knowing exit codes, options such as errexit
, and trap
enables us to construct robust scripts and manage problems with bash more effectively.
Exit Codes in Bash
Handling errors based on exit codes is the standard technique for detecting command failure. This is particularly true regarding external commands.
The curl
command in bash is an excellent example of handling problems based on known error codes. In contrast to user-defined routines, external command error codes are fully documented.
${?}
stores an exit status of the last command executed before a given line. A code of 0
indicates that the command was executed successfully. Otherwise, something went wrong.
case {?} in
0) {
true # ok
} ;;
*) {
false # something went wrong
}
Bash error handling might be performed with simple exit codes. You could attempt till you find a lazier solution. At the very least, any individual would do this after implementing a few conditionals to handle problems according to error codes.
Exit on Error
Exit on error is unquestionably the most helpful error detection and handling capability that bash programmers do not begin with.
trap
, EXIT
and ERROR
trap
permits us to specify commands to execute if the shell gets a signal. SIGNAL SPECs
are the names given to signals. Some frequent EXIT, ERROR, DEBUG, and RETURN
signals.
We can list more signals using the trap -l
command. We can also determine which commands are linked with a certain signal using trap -p SIGSPEC
.
For instance, we may wish to determine which commands correspond to the ERR
signal. In that situation, we could type the following command line:
trap -p ERR
If the output is empty, trap
has not yet linked any commands with the signal.
_() { echo oops ; }
trap _ ERR EXIT
Now, printing signal commands do not produce an empty result.
INPUT:
trap -p EXIT ERR
Output:
trap -- '_' EXIT
trap -- '_' ERR