How to Echo New Line in Bash
Fumbani Banda
Feb 02, 2024
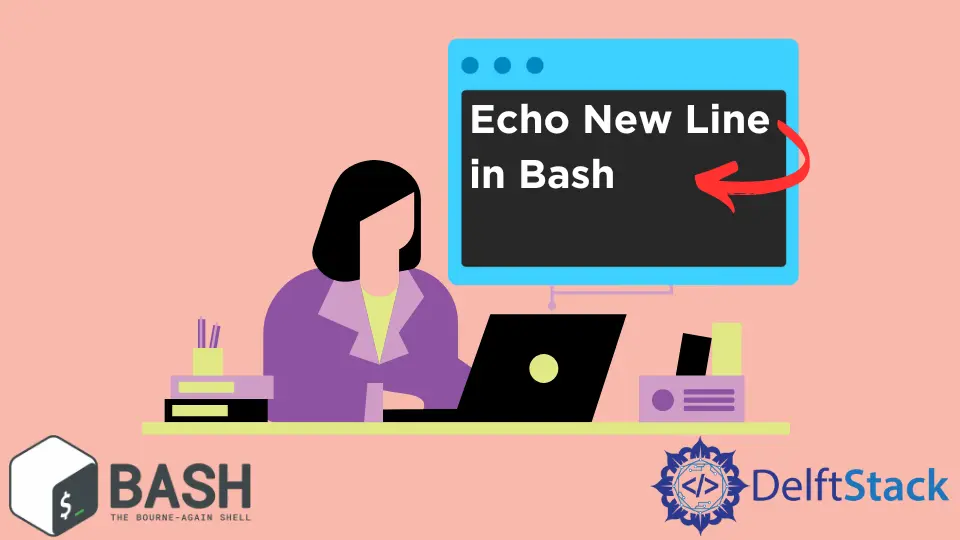
This tutorial will show the different ways to print a new line in bash using -e
and $
with the echo
command.
Bash echo
command
Bash echo
command is a command that is used to print output to the terminal.
echo 'I love working in Linux'
Output:
I love working in Linux
Echo New Line in Bash With -e
The echo
command does not recognize the new line character by default. To print a new line in bash, we need to enable the echo
command to interpret a new line character by adding the -e
option.
Using echo
to print a new line with the -e
option may not work on all systems. In some systems, the -e
option may be ignored. A better way to print a new line is to use printf
.
echo 'This is the first line \nThis is the second line'
Output:
This is the first line \nThis is the second line
echo -e 'This is the first line \nThis is the second line'
Output:
This is the first line
This is the second line
Echo New Line in Bash With $
We can use the $
before the new line character, which is inside single quotes, to print a new line with echo.
echo This is the first line$'\n'This is the second line
Output:
This is the first line
This is the second line
Author: Fumbani Banda