Difference Between $@ and $* in Bash Scripting
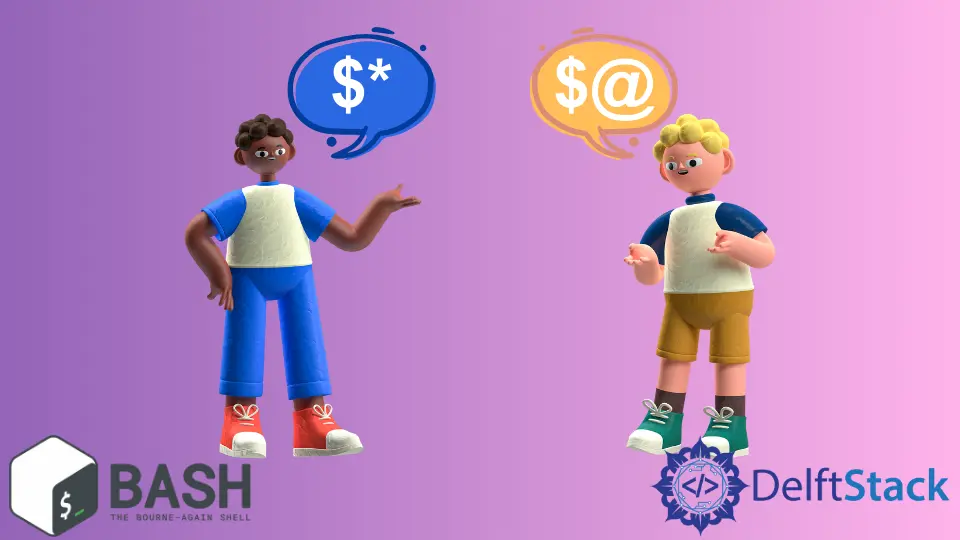
You can use the $@
and $*
to get the positional parameters in Bash scripting. This article will explain the difference between the asterisk ($*
) and at-sign ($@
) in Bash scripting.
Difference Between Asterisk ($*
) and At-Sign ($@
) in Bash Scripting
The $*
expression starts from one and expands to the positional parameters. If the expression is not used with double quotes, each positional parameter expands to a separate word.
#!/bin/bash
for i in $*; do
echo "* without quote -> $i"
done
If the expression is used with double quotes, the value of each parameter expands to a single word. The parameters are separated by the Internal Field Separator (IFS).
If the IFS is not defined, they are separated by a space. If it is set to null, the values are joined without intervening separators.
#!/bin/bash
for i in "$*"; do
echo "* with quote -> $i"
done
The $@
expression starts from one and expands to the positional parameters. If the expression is used with double quotes, each parameter expands to a separate word, but the parameters inside quotes are not split; that is, "$@"
is equivalent to $1 $2 …
.
#!/bin/bash
for i in "$@"; do
echo "@ with quote -> $i"
done
If the expression is used without double quotes, each parameter will expand into a separate word, including the parameters in quotes.
#!/bin/bash
for i in $@; do
echo "@ without quote -> $i"
done
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn