How to Create One Line Loop in Bash
- Using the For Loop
-
Using the
while
Loop - Using the Until Loop
- Using Command Substitution
- Conclusion
- FAQ
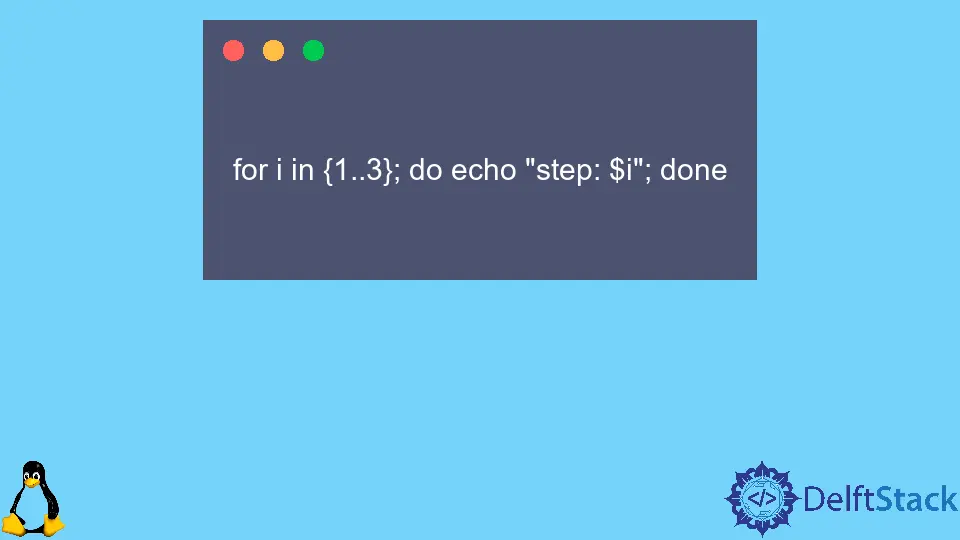
Creating loops in Bash can significantly streamline your scripting tasks, allowing you to automate repetitive actions with ease. Whether you’re a seasoned programmer or just starting with Bash scripting, knowing how to create one-line loops can enhance your productivity and efficiency.
In this article, we will explore various methods to create one-line loops in Bash, providing you with clear examples and detailed explanations. By the end, you’ll be equipped with the knowledge to implement these loops in your own scripts, making your workflow smoother and more effective.
Using the For Loop
The for
loop in Bash is one of the most straightforward ways to iterate over a list of items. You can create a one-line for loop that executes a command for each item in a specified list. Here’s how you can do it:
for i in {1..5}; do echo "Number: $i"; done
Output:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
In this one-liner, we use the for
loop to iterate through numbers 1 to 5. The do
keyword introduces the command to be executed for each iteration, which in this case is echo "Number: $i"
. The loop continues until all items in the specified range have been processed. This method is highly efficient for simple tasks and is easy to read, making it a popular choice for quick iterations.
Using the while
Loop
Another method to create a one-line loop in Bash is by using the while
loop. This type of loop continues executing as long as a specified condition is true. Here’s a compact example:
i=1; while [ $i -le 5 ]; do echo "Count: $i"; i=$((i + 1)); done
Output:
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
In this example, we initialize a variable i
to 1. The while
loop checks if i
is less than or equal to 5. If true, it executes the echo
command, printing the current count, and increments i
by 1. This method is particularly useful when the number of iterations is not predetermined, allowing for more flexibility in scripting.
Using the Until Loop
The until
loop is another powerful tool in Bash scripting that executes commands until a specified condition becomes true. This is useful in scenarios where you want to repeat actions until a certain state is achieved. Here’s an example:
i=1; until [ $i -gt 5 ]; do echo "Iteration: $i"; i=$((i + 1)); done
Output:
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Iteration: 5
In this one-liner, we start with i
set to 1 and use the until
loop to check if i
is greater than 5. The loop continues to execute the echo
command and increments i
until the condition is met. This approach is particularly effective when you want to ensure that a certain condition is not met before stopping the iterations.
Using Command Substitution
Command substitution allows you to execute a command and use its output as an argument in another command. You can also create one-line loops using this technique. Here’s how:
for file in $(ls); do echo "File: $file"; done
Output:
File: file1.txt
File: file2.txt
File: file3.txt
In this example, we use $(ls)
to list the files in the current directory, and the for
loop iterates over each file name. The echo
command then prints each file name prefixed with “File:”. This method is particularly useful for processing the output of commands dynamically, allowing for powerful scripting capabilities.
Conclusion
Creating one-line loops in Bash can greatly enhance the efficiency of your scripts. Whether you choose a for
, while
, or until
loop, each method has its unique advantages depending on your specific needs. By mastering these techniques, you can automate repetitive tasks, manipulate files, and streamline your workflow. With practice, you’ll find that these simple loops can lead to more complex and powerful Bash scripts, making your command-line experience much more productive.
FAQ
-
What is a one-line loop in Bash?
A one-line loop in Bash is a compact way to execute a loop structure, such asfor
,while
, oruntil
, in a single line of code. -
Can I use one-line loops for complex tasks?
Yes, while one-line loops are typically used for simpler tasks, they can be combined with other commands for more complex operations. -
Are there any limitations to using one-line loops?
One-line loops can be less readable for complex logic, making them harder to debug. It’s essential to balance brevity with clarity. -
Is it possible to nest one-line loops in Bash?
Yes, you can nest one-line loops, but be cautious, as this can lead to reduced readability and increased complexity. -
How can I learn more about Bash scripting?
Numerous online resources, tutorials, and documentation are available to help you learn more about Bash scripting and its capabilities.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn