How to Compare Numbers in Bash
Fumbani Banda
Feb 02, 2024
Bash
-
Use Square Braces
[]
to Compare Numbers in Bash -
Use Double Parenthesis
(( ))
to Compare Numbers in Bash
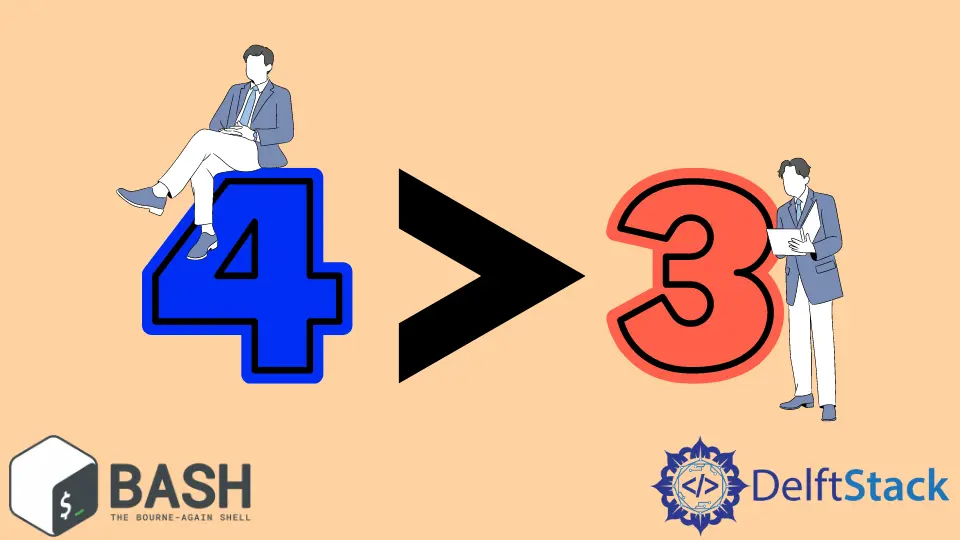
This tutorial will compare numbers in bash using square braces []
and double parenthesis - (( ))
.
Use Square Braces []
to Compare Numbers in Bash
The comparison operators must be used within the square braces.
x=4
y=3
if [ $x -eq $y ];
then
echo $x and $y are equal
elif [ $x -gt $y ]
then
echo $x is greater than $y
else
echo $x is less than $y
fi
Output:
4 is greater than 3
Use Double Parenthesis (( ))
to Compare Numbers in Bash
The comparison operators must be used within the double parenthesis.
x=4
y=10
if (( $x < $y ))
then
echo $x is less than $y
elif (( $x > $y ))
then
echo $x is greater than $y
else
echo $x is equal to $y
fi
Output:
4 is less than 10
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Fumbani Banda