How to Check Number of Arguments in Linux Bash
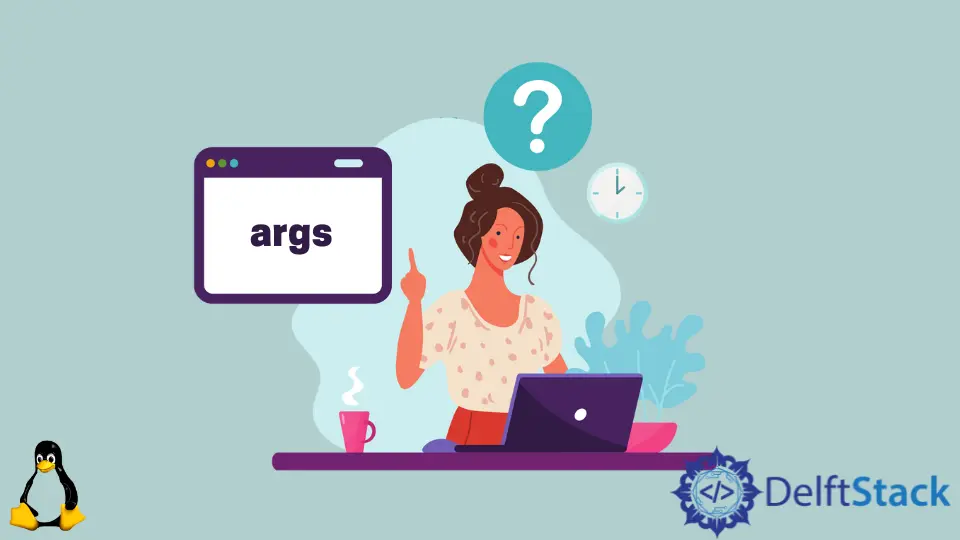
Bash script may need a certain number of command-line arguments to run. Throughout this article, we will explain how to use command-line arguments in Linux Bash. We will also clarify how to check the number of arguments.
Get Command Line Arguments in Bash
When executing a bash script, any value after the program name is given as an argument to the program. We have an example of how we can get these arguments.
The contents of the example.sh
file are below.
echo "Program name: $0"
echo "First argument: $1"
echo "Second argument: $2"
Let’s run the program and see the result.
./example.sh hello world
As you can see, the program name is the zeroth argument. Every value after the program name is taken sequentially.
Get Values of All the Arguments in Bash
We can use the $*
or the $@
command to see all values given as arguments.
echo "Args with *: $*"
echo "Args with @: $@"
This code prints all the values given as arguments to the screen with both commands.
Check Number of Arguments in Bash
We can use the $#
command to find the number of values given as arguments to the bash command. For example, we can use this command to prevent the program from executing without the required number of arguments.
if [ "$#" != "2" ]; then
echo "You must provide two args."
else
echo "Welcome!"
fi
This program will not continue to run unless it is executed with exactly two arguments.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn