How to Change Output Color of Echo in Bash
- Change the Foreground Color in Bash
- Change the Background Color in Bash
- Using the Escape Sequence in Bash
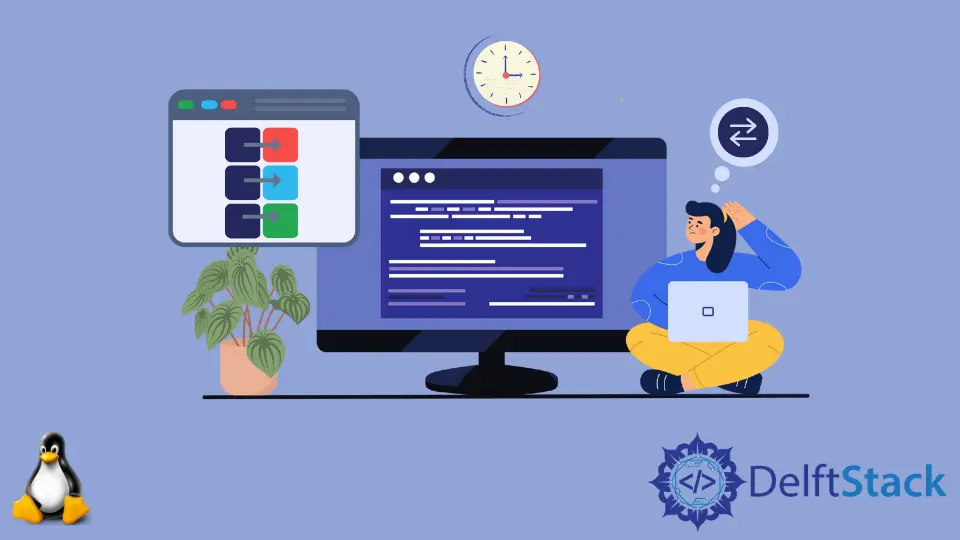
In this article, we will introduce different methods to change the colors of echo in Linux.
We will initiate changing the output colors of echo in Linux. We will also see an example of how to change the output colors of the background in Linux.
Change the Foreground Color in Bash
We are now looking at an example of foreground color changes in Linux.
$ echo "$(tput setaf 1)Shirt red $(tput setaf 4) Pant blue"
Output:
setaf
is used for the foreground color. Number 1
is red, whereas number 4
is blue.
The color numbers are as follows:
Number | Colors |
---|---|
1 | Black |
2 | Red |
3 | Green |
4 | Yellow |
5 | Blue |
6 | Cyan |
7 | White |
Change the Background Color in Bash
We can also change the background colors of an echo
.
$ echo "$(tput setaf 3) $(tput setab 1)sunflower is yellow"
Output
For the background color, setab
is used. The output shows that the background color has changed to red
, whereas the text color remains yellowish.
Using the Escape Sequence in Bash
Colored text on the terminal can be generated using escape sequences by a script. Each color has different color codes.
Let us look at an example.
$ echo -e "\e[1;31m Red is red in color bcoz its red"
In the above example, -e
allows the echo command to consider the escape sequences in the string, \e
represents the beginning of the escape sequence.
We also use [1
to make the text bold, 31
is the color code for red, and m
represents the conclusion of the escape sequence.
Output
As seen in the output, we printed text in red by using an escape sequence.
Colors in the 30
to 39
range are for the foreground. If we wish to modify the background color, we should utilize colors from 40
and above codes.
Following are the number for colors:
Color | Foreground | Background |
---|---|---|
Black | 30 | 40 |
Red | 31 | 41 |
Green | 32 | 42 |
Yellow | 33 | 43 |
Blue | 34 | 44 |
Magenta | 35 | 45 |
Cyan | 36 | 46 |
White | 37 | 47 |