How to Declare and Utilize Booleans in Bash
Nilesh Katuwal
Feb 02, 2024
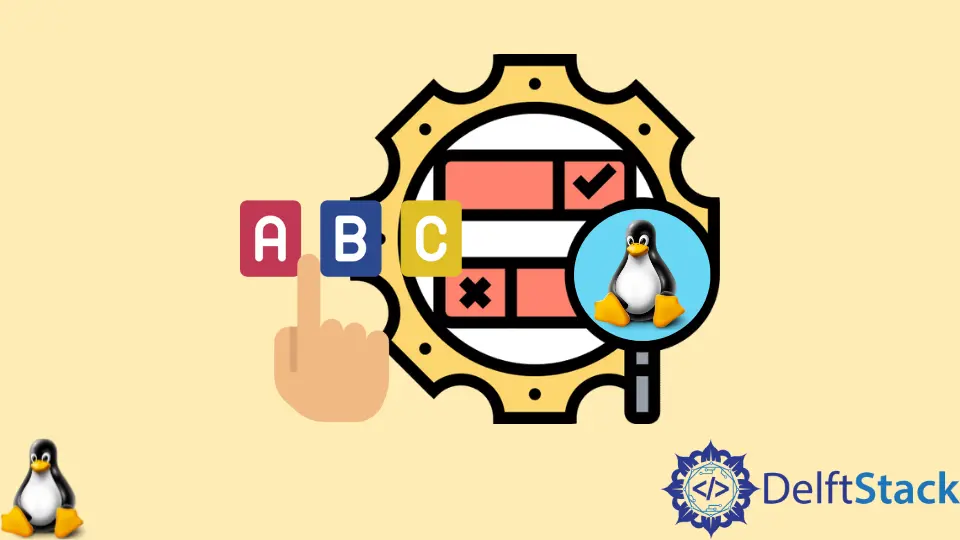
In Bash, there are no Booleans. However, we may specify the shell variable with values of 0
or False
and 1
or True
based on our requirements. Bash, on the other hand, supports logical boolean operators. It is required when the script must create output based on a variable’s True
or False
value.
Bash Booleans
Let’s look at an example.
#!/bin/bash
sunny=true
if $sunny ; then
echo 'Its a hot day!'
fi
Output:
Its a hot day!
Save the above script and run in terminal. Now, let’s change sunny
to false
.
#!/bin/bash
sunny=false
if $sunny ; then
echo 'Its a hot day!'
else
echo 'May be rainny!'
fi
Output:
May be rainny!
Using 0 or 1 as Booleans in Bash
Let’s look at an example. We have set sunny
to 0
and used -eq
to compare numerical values.
#!/bin/bash
sunny=0
if [ $sunny -eq 0 ]; then
echo 'Its a hot day!'
fi
Output:
Its a hot day!