How to Wait for Background Process in Bash
- Understanding Background Processes in Bash
- Using the wait Command
- Using Job IDs with wait
- Using wait with Exit Status
- Conclusion
- FAQ
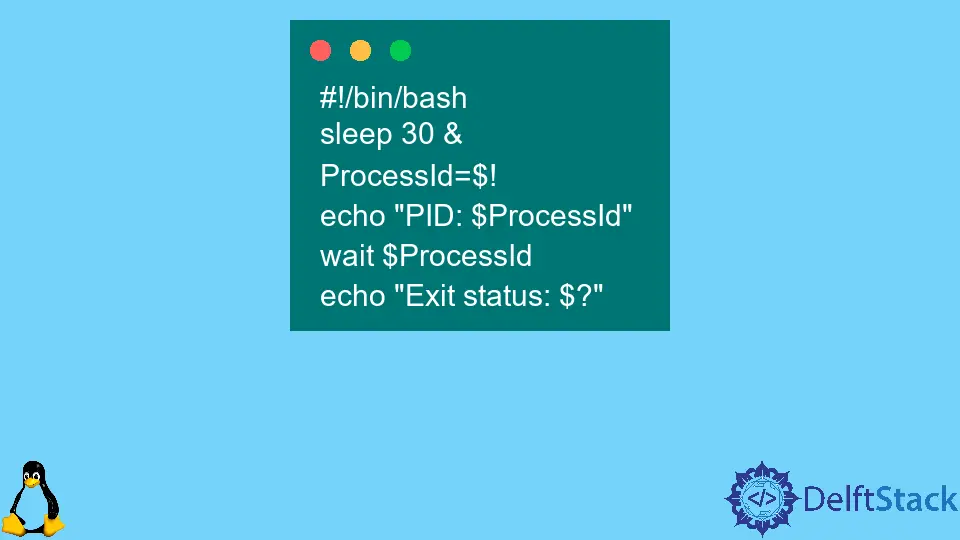
When working with Bash, managing background processes can be both powerful and tricky. Understanding how to wait for background processes is essential, especially if you’re leveraging these processes in a Git workflow.
In this tutorial, we will explore how to effectively wait for background processes in Bash, ensuring that your scripts run smoothly and efficiently. Whether you’re executing long-running Git commands or automating tasks, knowing how to handle background jobs will improve your productivity and script reliability. Let’s dive into the methods you can use to wait for these processes, and enhance your Bash scripting skills.
Understanding Background Processes in Bash
Before we jump into the methods for waiting on background processes, let’s clarify what a background process is. In Bash, when you run a command followed by an ampersand (&), it executes that command in the background. This allows you to continue using the terminal for other commands while the background task runs. However, sometimes you need to ensure that a background process has completed before proceeding. This is where the wait
command comes into play.
Using the wait Command
The simplest way to wait for a background process in Bash is to use the wait
command. This command waits for the specified background job to finish. If you don’t specify a job, it will wait for all background jobs to complete. This method is particularly useful when running multiple Git commands in parallel and you need to ensure they complete before moving on.
Here’s how you can use the wait
command:
#!/bin/bash
git clone https://github.com/example/repo.git &
git fetch &
git pull &
wait
echo "All Git commands executed successfully."
Output:
All Git commands executed successfully.
In this example, three Git commands are executed in the background. The wait
command is issued afterward, which pauses the script until all background processes are complete. This ensures that the final echo statement only runs once all Git operations have finished. Using wait
is straightforward and effective for managing multiple background processes.
Using Job IDs with wait
If you want to wait for a specific background process rather than all of them, you can use job IDs. When you run a command in the background, Bash assigns it a job ID. You can capture this ID and use it with the wait
command.
Here’s an example:
#!/bin/bash
git clone https://github.com/example/repo.git &
CLONE_PID=$!
git fetch &
FETCH_PID=$!
wait $CLONE_PID
echo "Clone completed. Now fetching..."
wait $FETCH_PID
echo "Fetch completed."
Output:
Clone completed. Now fetching...
Fetch completed.
In this script, we capture the process IDs of the background jobs using $!
. The first wait
command is used to pause until the clone operation is complete. After that, we fetch the changes, and the second wait
ensures that the fetch operation completes before moving on to the final echo statement. This method gives you more control over the execution flow of your background processes.
Using wait with Exit Status
Another useful feature of the wait
command is that it can return the exit status of the background process it waits for. This is particularly important when you want to check if a Git command succeeded or failed. By examining the exit status, you can make decisions in your script based on the success of the previous commands.
Here’s how you can implement this:
#!/bin/bash
git clone https://github.com/example/repo.git &
CLONE_PID=$!
wait $CLONE_PID
CLONE_STATUS=$?
if [ $CLONE_STATUS -eq 0 ]; then
echo "Clone successful."
else
echo "Clone failed with status $CLONE_STATUS."
fi
Output:
Clone successful.
In this script, after waiting for the clone process to finish, we capture its exit status in CLONE_STATUS
. The conditional statement checks if the exit status is zero (indicating success). If it is, we print a success message; otherwise, we indicate that the clone failed along with the exit status. This method is crucial for error handling in scripts, especially when working with version control systems like Git.
Conclusion
Waiting for background processes in Bash is a vital skill for anyone looking to streamline their scripting and automation efforts. By utilizing the wait
command, along with job IDs and exit statuses, you can effectively manage your background jobs, especially when working with Git commands. This not only enhances the reliability of your scripts but also helps in troubleshooting and error handling. With these techniques under your belt, you can take full advantage of Bash’s capabilities and improve your workflow.
FAQ
-
How does the wait command work in Bash?
The wait command pauses the execution of a script until the specified background process or all background processes complete. -
Can I wait for multiple background processes at once?
Yes, you can simply use the wait command without arguments to wait for all background processes to finish. -
What is the significance of job IDs in Bash?
Job IDs allow you to wait for specific background processes, providing more control over the execution flow in your scripts. -
How can I check if a background process was successful?
You can check the exit status of a background process using the $? variable after the wait command. -
Is it possible to run Git commands in the background?
Yes, you can run Git commands in the background by appending an ampersand (&) at the end of the command.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook