How to Assign One Variable to Another in Bash
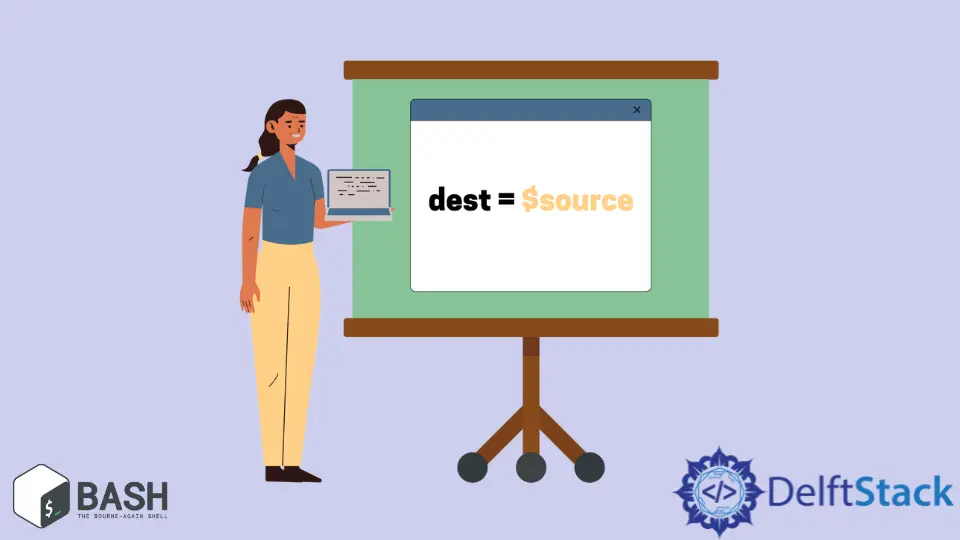
In Bash, a variable is created by giving its reference a value. Although the built-in declare statement in Bash is not required to declare a variable directly, it is frequently used for more advanced variable management activities.
To define a variable, all you have to do is give it a name and a value. Your variables should have descriptive names that remind you of their relevance. A variable name cannot contain spaces or begin with a number.
However, it can begin with an underscore. Apart from that, any combination of uppercase and lowercase alphanumeric characters is permitted.
Declare a Variable in Bash
To create a variable in the Bash shell, you must assign a value to that variable.
Syntax:
varname=value
Here, varname
is the name of the newly created variable, and value
is the value assigned to the variable. A value
can be null.
Let’s look at an example.
$ me=superman
$ this_year=2022
Using the echo
command, we can see the value of a variable. Whenever you refer to the value of a variable, you must prefix it with the dollar symbol $
, as seen below.
$ echo $me
Output:
superman
Let’s put all of our variables to work at the same time.
echo "$me $this_year"
Output:
superman 2022
Assign One Variable to Another in Bash
We can run dest=$source
to assign one variable to another. The dest
denotes the destination variable, and $source
denotes the source variable.
Let’s assign variable a
to variable b
.
$ a=Ironman
$ b=$a
$ echo "a=$b"
Output:
a=Ironman
Hence, we can easily assign the value of one variable to another using the syntax above.