How to Solve Syntax Error Near Unexpected Token in Bash
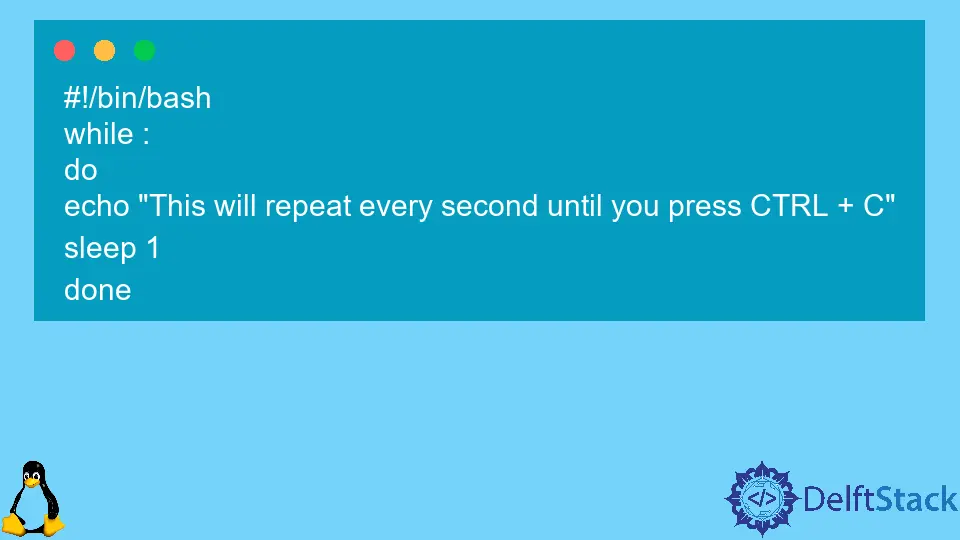
This article demonstrates identifying and correcting the unexpected token syntax errors caused due to the invisible characters in Bash scripts.
Syntax Error Near Unexpected Token in Bash
Sometimes, a Bash script that syntactically looks perfectly fine doesn’t work. You get a vague syntax error
that doesn’t help either.
Let’s say you have some code like:
#!/bin/bash
while :
do
echo "This will repeat every second until you press CTRL + C"
sleep 1
done
Syntactically, the above code is correct. However, some invisible characters may make the code syntactically unreadable in Linux.
How can we confirm whether the invisible characters are causing the problem or not? How do we solve that?
The solution is simple, as discussed in the preceding sections.
Identify the Problem
Assume that filename.sh
is the file you have saved for the Bash script.
Open your terminal, and navigate to the file location. Then, run the following command in the terminal.
cat -v filename.sh
The invisible characters, which are likely to represent carriage return or no-break space, should appear as ^M
, M-BM-
, or M-
. If any other weird characters get into your file, you should be able to see them as well.
We have a couple of options to fix our problem in our case.
Remove Windows Line Breaks Using Console Commands
If you have a problematic file with the name filename.sh
, we can use the following command to save the corrected contents into a file named correctedFile.sh
.
tr -d '\r' < filename.sh > correctedFile.sh
Automatically Convert Non-UNIX File Into a UNIX-Friendly Format
For this, you will need a utility called dos2unix
. If you are using apt
as your package manager, you can use the following command to install dos2unix
:
sudo apt install dos2unix
Or, if you are using an arch-based distribution, you can install dos2unix
from the AUR.
To convert the file into a UNIX-friendly format, open your terminal, and navigate to the folder containing your desired file. We will consider a file called filename.sh
.
Enter the following command in your terminal.
dos2unix filename.sh
The above command will convert your file into a UNIX-friendly format. Thus, solving the problem.
Hopefully, your Bash script runs fine now. If it’s still not running, you most likely have actual syntax problems.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn