How to Solve Syntax Error Near Unexpected Token in Bash
- Understanding the Syntax Error
- Common Causes of Syntax Errors
- Check for Missing Quotes
- Verify Command Structure
- Remove Extra Characters
- Check Parentheses Placement
- Conclusion
- FAQ
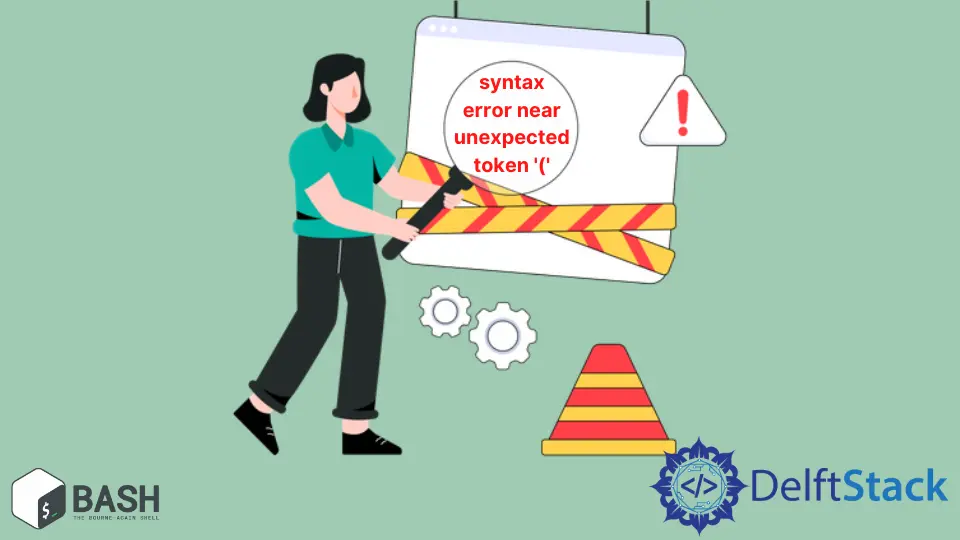
When working with Bash commands, encountering a syntax error near an unexpected token can be a frustrating experience. This error typically arises when the Bash interpreter encounters a command or symbol that it doesn’t expect. Whether you’re writing scripts or running commands in the terminal, understanding how to troubleshoot and resolve this issue is crucial for a smooth workflow.
In this article, we will explore common causes of syntax errors in Bash and provide effective solutions. By the end, you’ll be equipped with the knowledge to fix these errors confidently and enhance your command line proficiency.
Understanding the Syntax Error
Before diving into solutions, it’s essential to understand what a syntax error is. In Bash, a syntax error occurs when the shell cannot interpret a command due to incorrect formatting or unexpected characters. This can happen for various reasons, such as missing quotes, incorrect command structure, or even misplaced parentheses. Identifying the root cause is the first step toward resolving the issue.
Common Causes of Syntax Errors
Several factors can lead to syntax errors in Bash. Here are some common culprits:
- Missing Quotes: Forgetting to close a string with quotes can confuse the interpreter.
- Improper Command Structure: Commands must follow a specific syntax, and deviations can lead to errors.
- Extra Characters: Unintended characters or symbols can trigger unexpected token errors.
- Misplaced Parentheses: In Bash, parentheses have specific uses, and placing them incorrectly can result in syntax issues.
Now that we understand the common causes, let’s explore how to fix these errors.
Check for Missing Quotes
One of the most frequent causes of syntax errors in Bash is missing quotes. When you open a quote but forget to close it, Bash will continue to look for the closing quote, leading to confusion and errors.
Here’s an example of a command that would generate a syntax error due to missing quotes:
echo "Hello, World
Output:
bash: unexpected EOF while looking for matching `"'
bash: syntax error: unexpected end of file
In this example, the error message indicates that Bash is looking for a closing quote. To fix this error, simply ensure that every opening quote has a corresponding closing quote:
echo "Hello, World"
Output:
Hello, World
By carefully checking your quotes, you can avoid this common pitfall. Always ensure that strings are properly enclosed, especially when they contain spaces or special characters.
Verify Command Structure
Another frequent source of syntax errors is improper command structure. Bash commands must adhere to specific formatting rules. For instance, if you mistakenly place a command in the wrong order or forget to include necessary arguments, you may encounter an unexpected token error.
Consider the following example where the command is incorrectly structured:
ls -l | grep "txt"
Output:
ls: cannot access 'grep': No such file or directory
In this case, the command is structured correctly, but if you had forgotten the pipe symbol (|
), it would have resulted in an error. To resolve this, always double-check the command format and ensure that you’re using the correct syntax for each command.
For instance, the correct command structure should look like this:
ls -l | grep "txt"
Output:
file1.txt
file2.txt
By verifying the command structure, you can significantly reduce the chances of encountering syntax errors.
Remove Extra Characters
Extra characters in your command can lead to syntax errors as well. This includes stray symbols or characters that don’t belong. For instance, if you accidentally include a semicolon (;
) where it’s not needed, Bash will throw an error.
Here’s an example of how extra characters can cause issues:
echo "Hello, World!;
Output:
bash: unexpected token ';'
To fix this error, simply remove the unnecessary character:
echo "Hello, World!"
Output:
Hello, World!
Always review your commands for any unintended characters before executing them. A simple typo can lead to frustrating errors.
Check Parentheses Placement
When using parentheses in Bash, it’s crucial to place them correctly. Parentheses are used for grouping commands or creating subshells, and incorrect placement can lead to syntax errors.
Here’s an example of a command that generates a syntax error due to misplaced parentheses:
if (test -f "file.txt") then
echo "File exists"
fi
Output:
bash: syntax error near unexpected token 'then'
The correct structure requires the then
keyword to be on the same line or properly formatted. Here’s the corrected version:
if test -f "file.txt"; then
echo "File exists"
fi
Output:
File exists
By ensuring parentheses are correctly placed, you can avoid syntax errors associated with conditional statements and loops.
Conclusion
Syntax errors near unexpected tokens in Bash can be frustrating, but with a little understanding and attention to detail, they can be resolved effectively. By checking for missing quotes, verifying command structure, removing extra characters, and ensuring proper parentheses placement, you can significantly reduce the likelihood of running into these errors. Remember, practice makes perfect. The more you work with Bash, the more comfortable you’ll become in troubleshooting and resolving syntax issues. Happy coding!
FAQ
-
What does a syntax error near unexpected token mean?
A syntax error near unexpected token indicates that Bash encountered a command or character it didn’t expect, often due to formatting issues. -
How can I identify the cause of a syntax error in Bash?
Review your command for common issues such as missing quotes, improper structure, extra characters, or misplaced parentheses. -
Can syntax errors occur in scripts as well as in the terminal?
Yes, syntax errors can occur in both Bash scripts and commands run directly in the terminal. -
What should I do if I can’t find the syntax error?
Try breaking down your command into smaller parts to isolate the issue or consult online resources for syntax references.
- Is there a way to prevent syntax errors in Bash?
While you can’t eliminate them entirely, careful programming practices, thorough testing, and using a code editor with syntax highlighting can help minimize errors.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn