How to Check Syntax in Bash
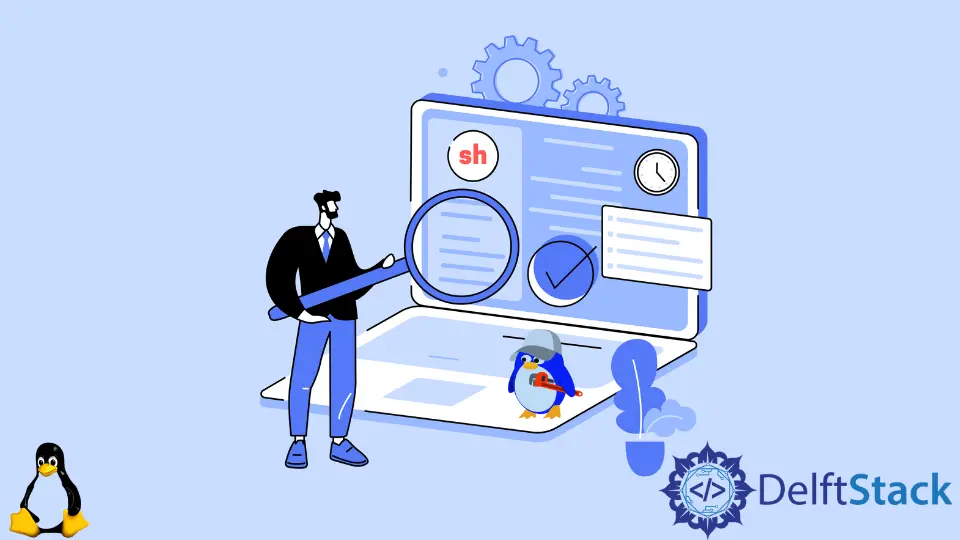
This article discusses ways to check a Bash script for syntactical issues without running the script.
Syntax errors occur due to some grammatical or typo errors in the code. In the modern compilers of other programming languages, such errors are highlighted by compilers even before running the program.
This is not the case in Bash scripts because Bash is a command-line interpreter. Therefore, we need to check for the syntax errors separately.
There can be different techniques for checking syntactical issues like the below.
Use Online ShellCheck
Editor
There is an online tool, Shell Check, to check the script for syntax errors. The tool can be found here.
In the image above, there are two separate windows. One is the code editor, and the lower part shows the list of errors and warnings in your code by specifying line numbers.
The ShellCheck
editor is a very helpful tool for beginners and expert programmers because it detects minor to advanced issues in the code before executing it.
Use the bash -n
(noexec
Mode) Command
You can also use the -n
flag (noexec
) with the bash
command to check for errors in the script.
bash -n [script-name]
This will just read all the commands in the script and check for errors without running them. If there are errors in the commands, it will show them; otherwise, no output will be displayed.
For example, consider the following script.
Note that we have missed the closing of the if
statement (i.e., used fi
instead of if
). So, it will give the error.
Use the sh
Command
Another command to check for syntax errors is:
sh -n [script-name]
We will run the command for the same script discussed in the previous section and check for errors.