Parameter Substitution in Bash
- Use Parameter Substitution in Linux Bash
- Remove Expression From String in Bash
- Replace Expression in String in Bash
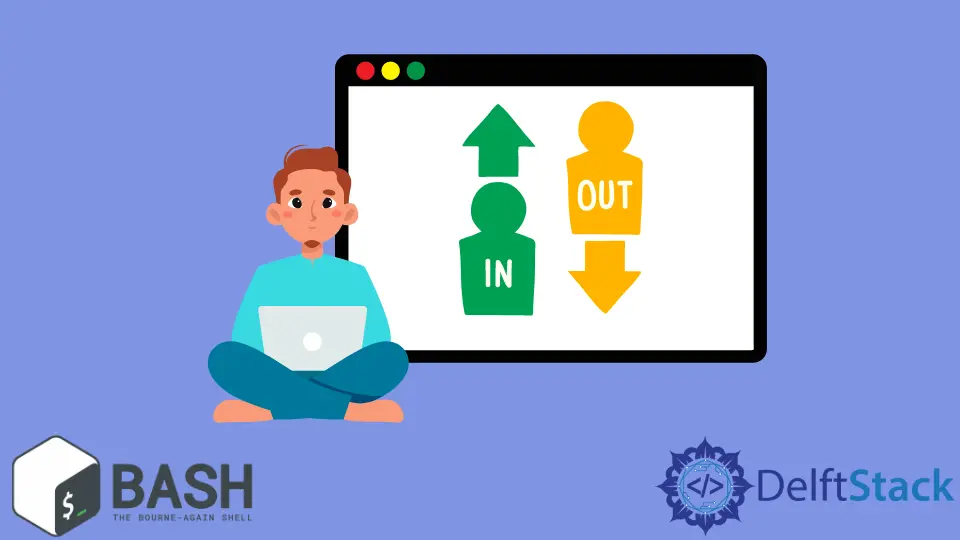
Sometimes we may want to delete a value from a string or replace it with a new one. There are many different ways to do this.
This article will explain how to use the parameter substitution method in Linux Bash scripting for this purpose.
Use Parameter Substitution in Linux Bash
Bash parameter substitution is an excellent way to remove or replace values from a string. The rest of the article will explain how we can do them with examples.
The following string will be used for examples.
str="one two three two one"
Remove Expression From String in Bash
There are two methods for deleting the expression from a string with the parameter substitution.
The ${string%expression}
command removes the expression
at the end of the string
. The last one
in the str
will be deleted in the example below.
echo "${str%one}"
The ${string#expression}
command removes the expression
at the beginning of the string
. The first one
in the str
will be deleted in the following example.
echo "${str#one}"
Replace Expression in String in Bash
The ${string/expression/value}
command replaces the first expression
it finds in the string
with the value
. The first one
in the str
will be replaced with four
in the example below.
echo "${str/one/four}"
The ${string//expression/value}
command replaces all the expression
it finds in the string
with the value
. In the following example, all the one
words in the str
will be replaced with the four
.
echo "${str//one/four}"
The ${string/#expression/value}
command replaces the expression
at the beginning of the string
with the value
. The first one
in the str
will be replaced with four
in the example below.
echo "${str/#one/four}"
The ${string/%expression/value}
command replaces the expression
at the end of the string
with the value
. The last one
in the str
will be replaced with four
in the following example.
echo "${str/%one/four}"
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn