How to Sort Array in Bash
- Use Bubble Sort to Sort Array in Bash
-
Use the
sort
Command to Sort Array in Bash -
Use the
readarray
Keyword to Sort Array in Bash - Conclusion
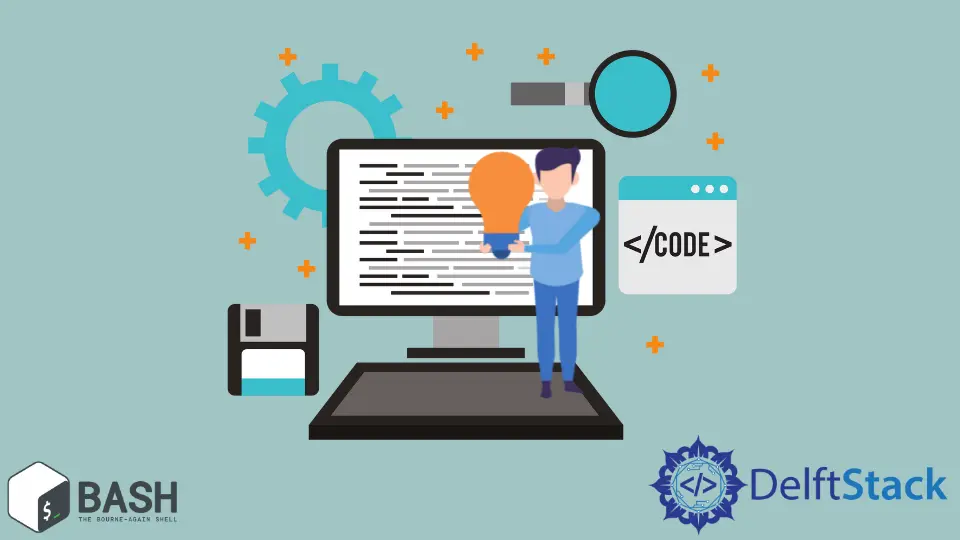
In this guide, we’ll explore various methods to efficiently perform a bash sort array operation, a key skill for any Bash scripting enthusiast.
Sorting arrays is a very common task for any programming language. In a Bash script, we also can do this task in two different ways.
We will explore various methods to efficiently achieve sorted values in arrays. The first one uses any sorting algorithms, and the second uses a built-in keyword in Bash script named readarray
.
Also, we will see some examples with explanations to make the topic easier.
Use Bubble Sort to Sort Array in Bash
One of the simplest sorting algorithms is the bubble sort. Its basic idea is to repeatedly step through the list, compare adjacent elements, and swap them if they are in the wrong order.
This process is repeated until the list is sorted.
Bubble sort has a straightforward concept, making it easy to implement in Bash, but it’s generally less efficient for large lists compared to more complex algorithms like quicksort or mergesort. However, for small datasets or for educational purposes, bubble sort is quite effective and illustrates basic sorting principles well.
Syntax and Parameters
In Bash, the bubble sort algorithm can be implemented using a nested loop. The outer loop tracks each pass through the array, and the inner loop compares adjacent elements and swaps them if necessary.
Key points in the syntax:
Outer Loop
: Runs as many times as there are elements in the array.Inner Loop
: Goes through the array, compares each pair of adjacent elements.Swap Logic
: If an element is greater than the next one, they are swapped.
The code for our example will be like the following.
#!/bin/bash
# Function to perform bubble sort
bubble_sort() {
local array=("$@")
local length=${#array[@]}
for ((i = 0; i < length; i++)); do
for ((j = 0; j < length-i-1; j++)); do
if [[ ${array[j]} > ${array[j + 1]} ]]; then
# Swap elements
temp=${array[j]}
array[j]=${array[j + 1]}
array[j + 1]=$temp
fi
done
done
echo "${array[@]}"
}
# Numeric array
numeric_array=(3 1 4 1 5 9 2 6 5 3 5)
echo "Sorted Numeric Array:"
bubble_sort "${numeric_array[@]}"
# String array
string_array=("apple" "orange" "banana" "grape" "cherry")
echo "Sorted String Array:"
bubble_sort "${string_array[@]}"
In this script, we first define a function bubble_sort
that performs the sorting on the array variable. This function takes an array as an argument and sorts it using the bubble sort algorithm.
We then use this function to sort two different types of arrays: numeric and string.
We use nested loops to compare each element with its next one and swap them if needed. The swapping is done using a temporary variable, a common technique in sorting algorithms.
The script finally prints the arrays as a sorted list using the echo
command. The usage of a function makes our script versatile and reusable for different types of arrays.
Output:
This output demonstrates the successful sorting of both numerical and string arrays. The numeric array is sorted in ascending numerical order, while the string array is sorted alphabetically.
This simple yet effective implementation of bubble sort in Bash is a valuable exercise in understanding basic sorting algorithms and their application in shell scripting.
Use the sort
Command to Sort Array in Bash
Sorting is a fundamental operation in programming, often required for organizing data, optimizing algorithms, or simply improving readability. In the shell language of Bash, the sort
command stands out as a powerful tool for this purpose.
This command, primarily designed for sorting lines in text files, can be ingeniously repurposed to sort arrays with ease and efficiency. This approach is particularly beneficial when dealing with large datasets, as sort
is optimized for performance and offers a variety of sorting options.
Syntax and Parameters of sort
in Bash
The sort
command in Bash is versatile, with several key parameters:
-n
: Sorts the input as numbers.-r
: Reverses the sorting order.-k
: Sorts based on a specified field or column.-t
: Defines a delimiter for separating fields.
In the context of array sorting, we often pipe the array elements into sort
using a command like printf
to format the array elements as lines, which sort
can then process.
Here’s an example that demonstrates how to sort an array using this command:
#!/bin/bash
# Sorting a Numeric Array
numeric_array=(3 2 5 4 1)
printf "%s\n" "${numeric_array[@]}" | sort -n
# Sorting a String Array
string_array=("dog" "cat" "elephant" "bear" "ant")
printf "%s\n" "${string_array[@]}" | sort
In our code, we first declare two arrays: numeric_array
and string_array
. To sort these arrays, we use printf "%s\n"
to print each element on a new line, which is then piped (|
) into the sort
command.
For the numeric array, we use sort -n
to ensure numerical sorting. For the string array, a simple sort
suffices, as it sorts alphabetically by default.
This approach is efficient and concise, leveraging sort
’s powerful sorting capabilities without the need for writing complex sorting algorithms in Bash.
Output:
The numeric array is sorted in ascending numerical order, while the string array is sorted alphabetically. This demonstration highlights the sort
command’s utility in Bash for array sorting, providing an efficient and straightforward solution suitable for both newcomers and experienced users in the realm of shell scripting.
The elegance of this method lies in its simplicity and the leveraging of an existing, well-optimized system command, making it an excellent choice for various sorting needs. While the sort
command is powerful, it’s not the answer for sorting multi-dimensional arrays or when array elements have complex structures.
Use the readarray
Keyword to Sort Array in Bash
Bash, a powerful scripting environment, offers various tools for data manipulation but lacks a direct array sorting function. This gap can be bridged by combining Bash commands in creative ways.
A particularly effective method is using readarray
in tandem with the sort
command. This approach is ideal for sorting arrays in Bash, especially when dealing with dynamically generated data or inputs from external sources.
The key purpose of using readarray
along with sort
in Bash is to harness the robust sorting features of sort
and seamlessly store the sorted results back into an array. This method is not just efficient, but it also enhances script readability and maintainability, catering to both novice and seasoned script writers.
Syntax and Parameters
The essential syntax to sort an array in Bash using readarray
and sort
is as follows:
sorted_output=$(printf "%s\n" "${array[@]}" | sort [options])
readarray -t sorted_array <<< "$sorted_output"
printf "%s\n"
: Outputs each element of the original array on a new line, preparing it for sorting.sort [options]
: Applies sorting to the input. Options like-n
can be used for numerical sorting.readarray -t sorted_array
: Captures the sorted output into an array. The-t
option trims trailing newline characters.
Here’s the code that demonstrates how to use the sort
command along with readarray
to sort the array:
#!/bin/bash
# Sorting a Numeric Array
numeric_array=(3 2 5 4 1)
sorted_numeric_output=$(printf "%s\n" "${numeric_array[@]}" | sort -n)
readarray -t sorted_numeric_array <<< "$sorted_numeric_output"
echo "Sorted Numeric Array: ${sorted_numeric_array[*]}"
# Sorting a String Array
string_array=("dog" "cat" "elephant" "bear" "ant")
sorted_string_output=$(printf "%s\n" "${string_array[@]}" | sort)
readarray -t sorted_string_array <<< "$sorted_string_output"
echo "Sorted String Array: ${sorted_string_array[*]}"
In this script, two arrays, numeric_array
and string_array
, are defined. Each array is sorted using the sort
command, with the sorted results being captured in a variable.
The readarray
command then reads this sorted data into a new array. Finally, we use the [*]
expansion to concatenate the sorted array elements for output.
This script exemplifies an efficient, readable way to handle array sorting in Bash.
Output:
This output demonstrates the script’s effectiveness in sorting both numeric and string arrays. The technique outlined here is a practical, easily understandable solution for array sorting in Bash scripts, enhancing the script’s ability to process and organize data efficiently.
Conclusion
Sorting an array is a common task in programming. In Bash scripting, the sort
command provides a straightforward and efficient way to achieve this.
By leveraging the sort
command and understanding its usage, a result of dedicated research effort, you can easily sort arrays and work with the data in the desired order. This article has provided a comprehensive guide and complete working example to help you understand how to effectively sort an array in Bash using the sort
command.
Please note that all the code used in this article is written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn