How to Solve Syntax Error Near Unexpected Token Newline in Linux Bash
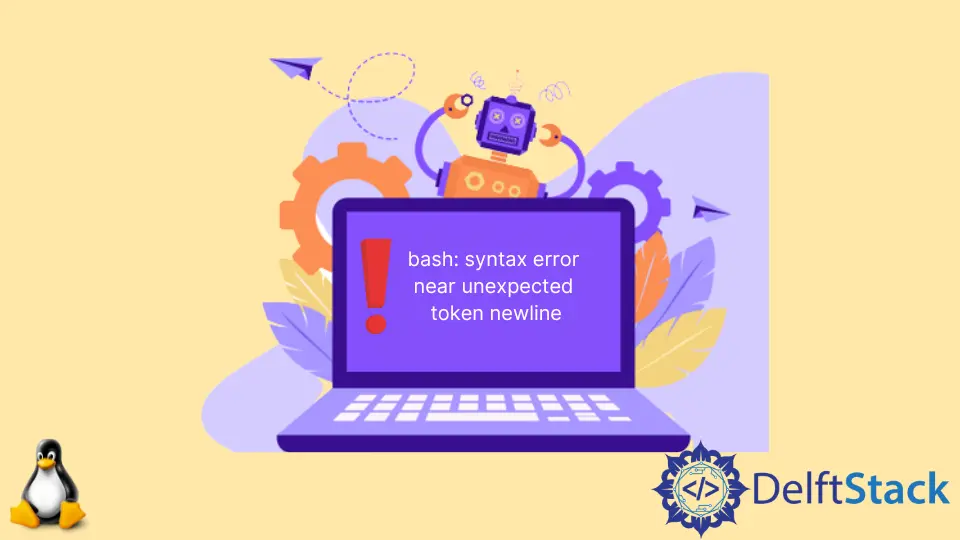
When writing code with Bash scripting, special characters or lack of quotation marks may cause errors. This article will explain how to solve the bash: syntax error near unexpected token newline
in Linux Bash.
Solve the bash: syntax error near unexpected token newline
Error in Linux Bash
There are many code-related reasons why you get a syntax error near unexpected token newline
error in Bash scripting. The rest of the article explains the most common mistakes and ways to fix them.
If you are getting this error, you most likely made a syntax error. First, read the file’s contents with the cat
command.
cat file.sh
Note the use of special characters. The <
character is used for input redirection, and the >
character is used for output redirection.
If you are using these angle brackets, you must use them in quotes. Otherwise, an error will occur.
For example, the following code will throw the syntax error near unexpected token newline
.
#!/bin/bash
str=<you cannot do this>
However, you can use the angle brackets with quotes. The code below will work without errors.
#!/bin/bash
str="<You can do this>"
It does not matter if you use double quotes or single quotes. You can also do it as below.
#!/bin/bash
str='<You can also do this>'
Similarly, you cannot use angle brackets in the Bash command line parameters. Let’s examine the example below.
There is a login program that takes username and password as arguments. If any of the arguments contain an angle bracket, you will get a bash: syntax error near unexpected token newline
error.
./login --username admin --password 1234<
Enclose the argument in single or double quotes to avoid an error.
./login --username admin --password "1234<"
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn