How to Set -e in Bash Scripting
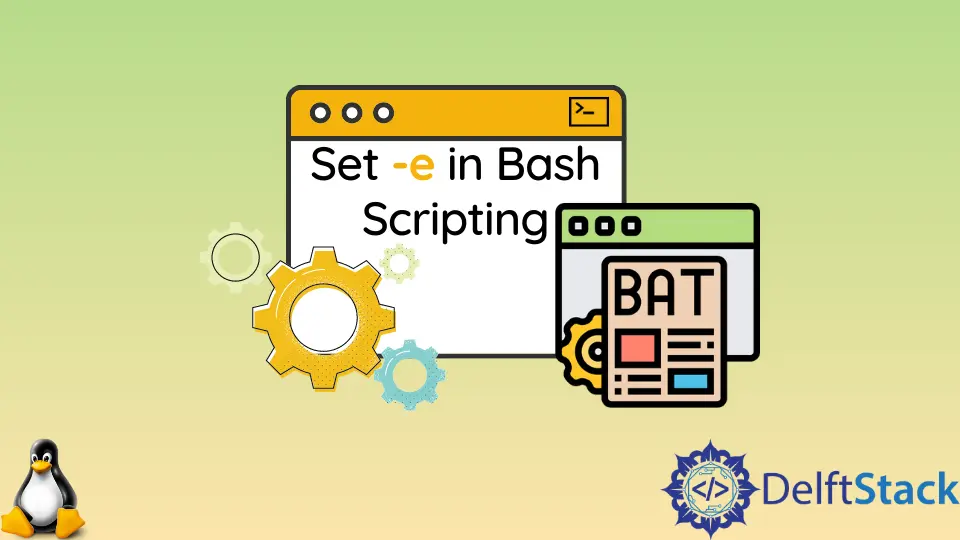
If the command returns a non-zero exit status, the set -e
option instructs the shell to exit. In simple terms, the shell exits when a command fails.
In Debian package management scripts, set -e
is also known as set -o errexit
. According to Debian policy, the usage of this option is required in certain scripts; the goal appears to be to avoid any chance of an unhandled error scenario.
In reality, this means that you must understand the situations under which the commands you run may return an error and explicitly address each of those problems.
Using set -e
Outside the Function Body in Bash
Let’s write a Bash script to print Hello World
using the hello_world
function. We’ll use set -e
outside of the function.
set -e
is only used to write or set an error displaying code.
#!/bin/bash
set -e
function hello_world()
{
echo "Hello World!"
}
hello_world
Output:
Hello World!
Using set -e
Inside the Function Body in Bash
Now, we’ll put set -e
inside the function in the above script.
#!/bin/bash
function hello_world()
{
set -e
echo "Hello World!"
}
hello_world
Output:
Hello World!
Using set-e
inside or outside a function does not affect our output.
set -e
With trap
The set -e
command instructs the terminal to throw an exception when it detects an error, causing the code to halt execution. The trap
keyword allows you to utilize a built-in function, ERR
, which accepts the error line number and passes it to the error function.
Let’s look at an example.
#!/bin/bash
set -e
function error()
{
echo "error at $1"
}
trap 'error $LINENO' ERR
errors
We made a function error
and used the shell variable $1
to store the line number where the error occurs.
Output:
demo.sh: line 9: errors: command not found
error at 9
demo.sh
is the name of the script file that contains the above script.
If one line of a script fails, but the last line succeeds, the script as a whole is considered successful. As a result, it is quite easy to overlook the error.
Being error-intolerant is much better in scripts, and set -e
provides you with that.