How to Add New Users in Linux in Bash Script
- Understanding the Basics of User Management in Linux
- Creating a Simple Bash Script to Add Users
- Enhancing the Script with Additional Features
- Conclusion
- FAQ
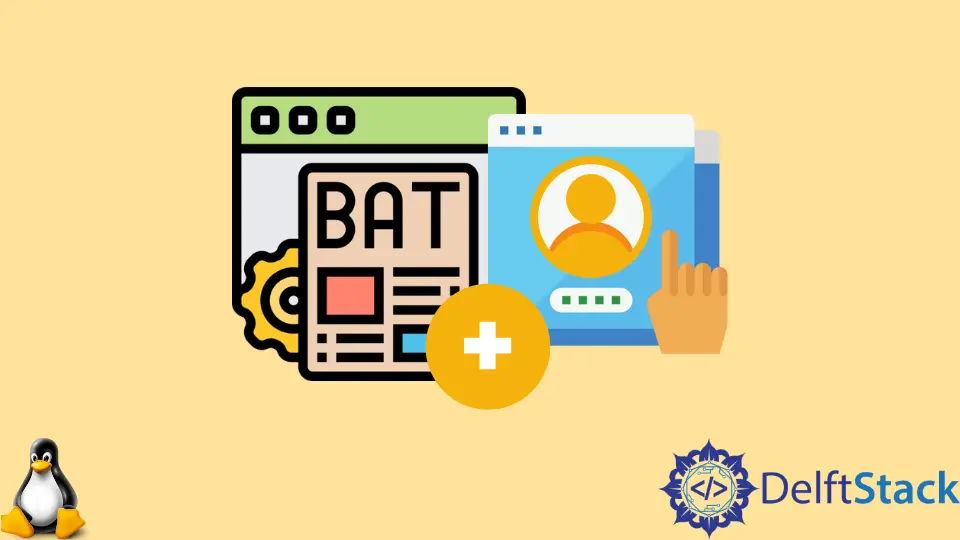
Adding new users in Linux can be a straightforward task, especially when you use a Bash script to automate the process.
This tutorial will guide you through creating a simple yet effective Bash script that can add users to your Linux system with ease. Whether you’re managing a server or just want to streamline your user management tasks, this guide will provide you with the tools you need. By the end of this article, you’ll be able to write a Bash script that not only adds users but can also be customized to include additional parameters like setting passwords and user groups. Let’s dive into the world of Bash scripting and user management in Linux!
Understanding the Basics of User Management in Linux
Before we jump into the script, it’s essential to understand how user management works in Linux. The primary command for adding a user is useradd
. This command creates a new user account, but it does not set a password by default. You can also specify various options to customize the user account, such as the home directory and user group.
In a Bash script, you can automate the useradd
command, making it easier to add multiple users at once. The script will prompt you for the necessary details and execute the commands accordingly. This approach saves time and reduces the potential for errors.
Creating a Simple Bash Script to Add Users
Let’s start by creating a simple Bash script that will allow you to add users. Open your terminal and follow these steps:
-
Create a new file for your script. You can name it
add_users.sh
:touch add_users.sh
-
Open the file in your preferred text editor. For example, using
nano
:nano add_users.sh
-
Now, let’s write the script:
#!/bin/bash read -p "Enter username: " username sudo useradd -m "$username" echo "User $username has been added."
-
Save and exit the editor.
To run the script, you need to give it execute permissions:
chmod +x add_users.sh
Now you can execute the script:
./add_users.sh
Output:
Enter username: newuser
User newuser has been added.
This script prompts for a username, uses the useradd
command to create the user, and confirms the action. The -m
option creates a home directory for the user automatically.
Enhancing the Script with Additional Features
While the basic script works, you can enhance it with additional features, such as setting a password and adding users to specific groups. Here’s how you can do that:
-
Open your
add_users.sh
file again:nano add_users.sh
-
Modify the script to include password setting and group addition:
#!/bin/bash read -p "Enter username: " username read -sp "Enter password: " password echo read -p "Enter user group (leave blank for default): " group sudo useradd -m -p "$(openssl passwd -1 "$password")" "$username" if [ -n "$group" ]; then sudo usermod -aG "$group" "$username" fi echo "User $username has been added."
- Save and exit the editor.
Now, when you run the script, it will prompt you for a username, password, and an optional group. The openssl passwd -1
command encrypts the password before adding the user, enhancing security.
Output:
Enter username: newuser
Enter password:
Enter user group (leave blank for default): developers
User newuser has been added.
This enhanced script not only creates a user but also sets a password and adds the user to a specified group if provided. This flexibility is particularly useful in larger environments where user roles and permissions need careful management.
Conclusion
Creating a Bash script to add users in Linux can significantly simplify user management tasks. By following this tutorial, you learned how to write a basic script and enhance it with additional features like password setting and group assignments. This automation not only saves time but also minimizes errors that can occur when adding users manually. Whether you’re managing a small team or a large organization, having a reliable script at your disposal can make a world of difference. Happy scripting!
FAQ
-
How do I run a Bash script in Linux?
You can run a Bash script by navigating to its directory in the terminal and using the command./script_name.sh
, ensuring the script has execute permissions. -
Can I add multiple users at once using a Bash script?
Yes, you can modify the script to accept a list of usernames and loop through them to create multiple users in one execution. -
What does the
-m
option do in theuseradd
command?
The-m
option creates a home directory for the new user automatically. -
How can I check if a user already exists before adding them?
You can use theid
command to check if a user exists and conditionally add them in your script. -
Is it safe to use plain text for passwords in scripts?
No, it is not safe to use plain text passwords. Always encrypt passwords before storing or using them in scripts.