How to Get Bash Script Filename
-
Use the
basename
Command to Get Bash Script Filename - Use the Parameter Expansion to Get Bash Script Filename
-
Use the
BASH_SOURCE
Variable to Get Bash Script Filename
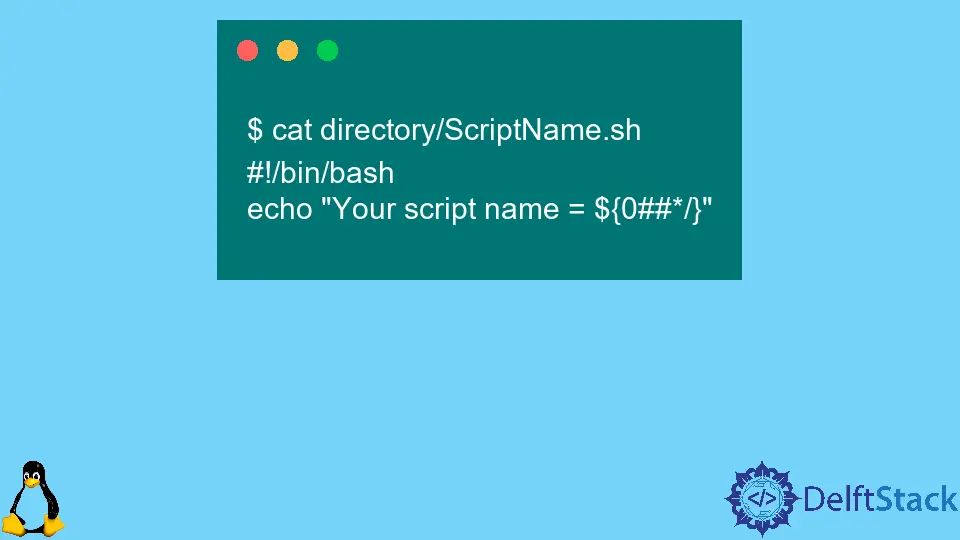
This article shows how we can get the filename of its own by the script. Also, we will see necessary examples and explanations to make the topic easier.
There are many ways available to do this task. But we are going to see three easy methods to do this.
Let’s take a look at them.
Use the basename
Command to Get Bash Script Filename
We can get the script’s filename by using the basename
command, a built-in command on Bash. To do this, you have to follow the below code.
$ cat directory/ScriptName.sh
#!/bin/bash
echo "Your script name =" $(basename "$0")
You can notice that we used the $0
with the basename
command to find the script’s filename. This $0
is a built-in variable in Bash that represents the filename of the relative path.
After executing the command, we will see the below output.
$ directory/ScriptName.sh
Your script name = ScriptName.sh
Use the Parameter Expansion to Get Bash Script Filename
We can also do the same task using parameter expansion. We need to follow the code below to do the same task with this method.
$ cat directory/ScriptName.sh
#!/bin/bash
echo "Your script name = ${0##*/}"
You can notice that we used some combination of symbols, ${0##*/}
, which is used to show the parameter expansion. After executing the command, we will see the below output.
$ directory/ScriptName.sh
Your script name = ScriptName.sh
Use the BASH_SOURCE
Variable to Get Bash Script Filename
BASH_SOURCE
is a built-in environment variable in Bash that contains the filename. To find the script’s filename using this variable, you need to follow the code below.
$ cat directory/ScriptName.sh
#!/bin/bash
echo "Your script name =" $(basename "${BASH_SOURCE}")
After executing the command, we will see the below output.
$ directory/ScriptName.sh
Your script name = ScriptName.sh
Please note that all the code used in this article is written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn