How to Remove Line From File in Bash
-
Use
tail
to Remove a Line of a Text File -
Use
sed
to Remove a Line of a Text File -
Use
awk
to Remove a Line of a Text File - Conclusion
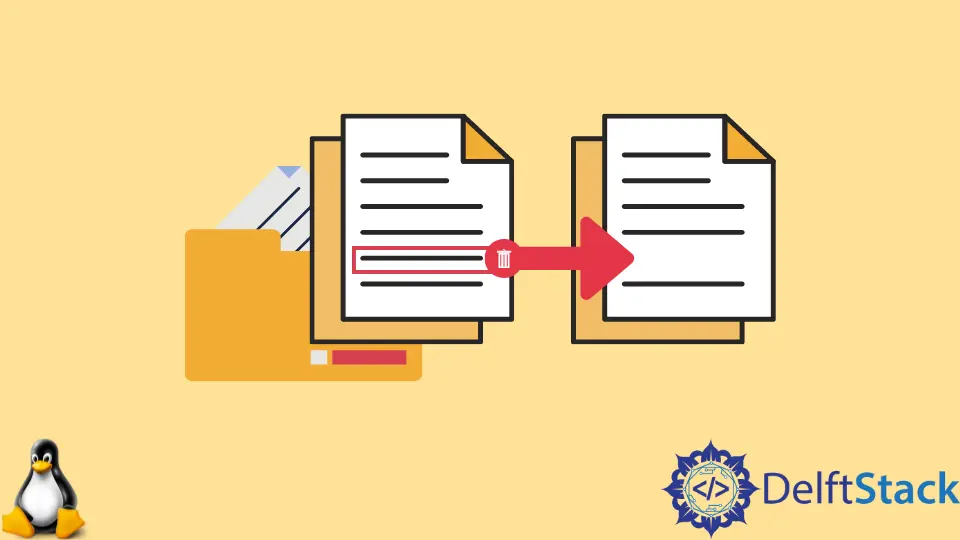
In a Bash script, here are several ways to eliminate a line from a file. This article will discuss different ways to remove unnecessary lines from a file.
Suppose we have a text file named Test.txt
with the contents below.
This is the first line.
This is the second line.
This is the third line.
This is the fourth line.
Use tail
to Remove a Line of a Text File
We can use the built-in keyword in Bash known as the tail
to remove unnecessary lines from our files.
Command:
tail -n +2 Test.txt
The -n +2
will print everything in the file except the first line. The -n +1
will print the whole file.
The +
sign inverts the argument and instructs the tail
to print everything.
Output:
This is the second line.
This is the third line.
This is the fourth line.
Use sed
to Remove a Line of a Text File
Another built-in command in Bash is named the sed
, a built-in Linux tool mainly used for text manipulation. The full form of the command is Stream editor, as this keyword takes the text in the form of a stream and performs numerous operations.
In our example below, we will delete the first line from the file.
Command:
sed '1d' Test.txt
The '1d'
instruct the sed
command to perform a delete action on the first line.
Output:
This is the second line.
This is the third line.
This is the fourth line.
Use awk
to Remove a Line of a Text File
In our example below, we will delete the first line from our file using awk
.
Command:
awk 'NR>1' Test.txt
The 'NR>1'
indicates the line number greater than 1
. It will show only the lines after the first line.
Output:
This is the second line.
This is the third line.
This is the fourth line.
Conclusion
We shared three different ways to remove a line from the file, and you can choose one based on your needs. Note that all the code used in this article is written in Bash and will only execute in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn