How to Read File Into Variable in Bash
- Text File to Save Into a Variable
-
Use the
cat
Keyword to Read File Into Variable in Bash -
Read File Into Variable in Bash Without Using the
cat
Keyword
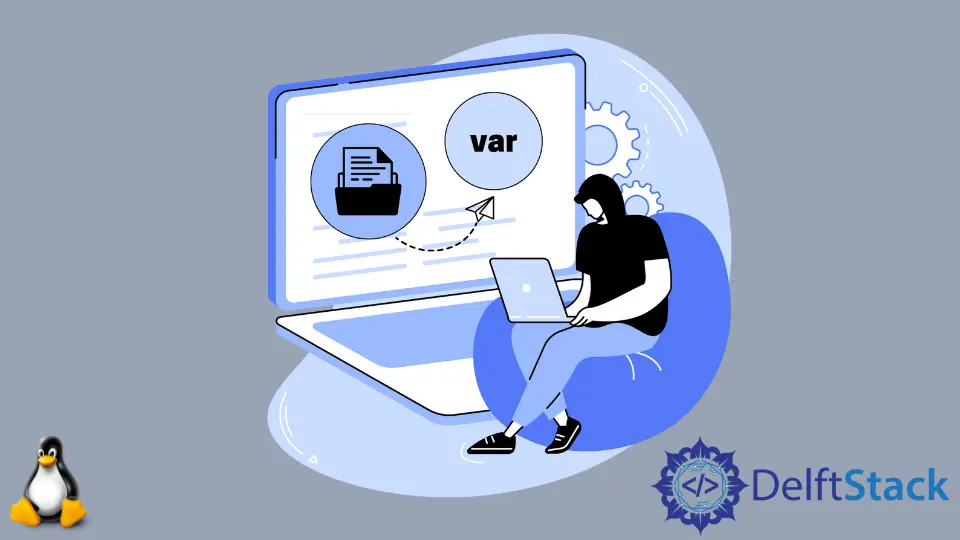
Sometimes when working with files, we may need to take the text file in a variable to perform some necessary operations on the file. In Bash Script, it is a very easy task and does need not more than two lines of code.
This article will show how we can take a file in a variable. Also, we will see necessary examples with proper explanations to make the topic easier.
Text File to Save Into a Variable
Before we start, suppose we have a text file with the following contents:
This is the first line.
This is the second line.
This is the third line.
This is the fourth line.
Now, we are going to use the below three methods one by one to take the file into a variable,
Use the cat
Keyword to Read File Into Variable in Bash
In our below method example, we will use a built-in keyword of Bash, cat
. This keyword is mainly used to read any file.
The code for our example will be like the below:
FileText= cat 1_Test.txt
echo "$FileText"
In the example above, we just read a text file using the cat 1_Test.txt
and assigned the data to a variable named FileText
, and after that, we print the data stored in the variable.
After executing the above Bash script, you will get an output like the following:
This is the first line.
This is the second line.
This is the third line.
This is the fourth line.
Read File Into Variable in Bash Without Using the cat
Keyword
This method will do the same task but won’t use the keyword cat
. You can follow the below example to take the text file in a variable.
FileText=$(<1_Test.txt)
echo "$FileText"
In the example above, we just read a text file using $(<1_Test.txt)
and assigned the data to a variable named FileText
. After that, we printed the data stored in the variable.
After executing the above Bash script, you will get an output like the following:
This is the first line.
This is the second line.
This is the third line.
This is the fourth line.
You can choose any one of the above methods based on your preferences.
Please note that all the code used in this article is written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn